My Developer Journal
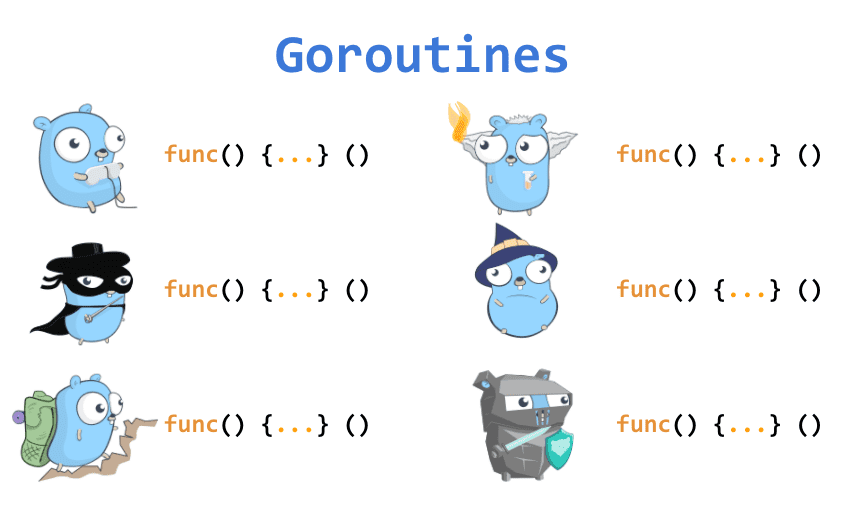
Comprehensive Course on Go Concurrency: Goroutines and Channels

Welcome to the comprehensive course on Goroutines and Channels in Go! This course is designed to take you from the basics to advanced concepts, ensuring you become an expert in Go's concurrency model. By the end of this course, you'll understand how to effectively utilize goroutines and channels to build efficient, concurrent applications in Go. Table of Contents Introduction to Concurrency in Go Goroutines: The Building Blocks of Concurrency Channels: Communication Between Goroutines Channel Operations Buffered vs. Unbuffered Channels Channel Directionality The select Statement Synchronization with WaitGroups Avoiding Common Concurrency Pitfalls Concurrency Patterns and Best Practices Advanced Topics 11.1 Context Package 11.2 Pipelines 11.3 Fan-In/Fan-Out 11.4 Worker Pools Practical Examples and Projects Conclusion and Next Steps 1. Introduction to Concurrency in Go What is Concurrency? Concurrency refers to the ability of a system to handle multiple tasks simultaneously. In programming, this means managing multiple processes or threads that can execute independently while potentially interacting with each other. Go's Approach to Concurrency Go provides built-in support for concurrency, making it easier to write programs that can perform multiple tasks simultaneously. Its concurrency model is based on goroutines and channels, which simplify the process of managing concurrent tasks compared to traditional threading models. Benefits of Concurrency Performance: Utilize multiple CPU cores to perform tasks in parallel. Responsiveness: Improve the responsiveness of applications by performing tasks concurrently. Resource Efficiency: Lightweight goroutines consume fewer resources compared to traditional threads. Concurrency vs. Parallelism Concurrency: Managing multiple tasks at the same time, potentially interleaving their execution. Parallelism: Executing multiple tasks simultaneously on multiple processors or cores. Go's concurrency model primarily focuses on concurrency, but it can leverage parallelism on multi-core systems. 2. Goroutines: The Building Blocks of Concurrency What are Goroutines? A goroutine is a lightweight thread managed by the Go runtime. Goroutines are functions or methods that run concurrently with other goroutines. Starting a Goroutine You can start a goroutine by using the go keyword followed by a function call. package main import ( "fmt" "time" ) func sayHello() { fmt.Println("Hello from goroutine!") } func main() { go sayHello() // Starts a new goroutine time.Sleep(1 * time.Second) // Wait to allow goroutine to finish fmt.Println("Hello from main!") } Output: Hello from goroutine! Hello from main! Key Characteristics of Goroutines Lightweight: Goroutines have a small initial stack (around 2KB) that grows and shrinks dynamically, allowing thousands of goroutines to run concurrently. Managed by Go Runtime: The Go scheduler multiplexes goroutines onto OS threads, abstracting away the complexity of thread management. Concurrent Execution: Goroutines execute independently and can communicate via channels. Example: Goroutines with Anonymous Functions Goroutines can also be started with anonymous functions. package main import ( "fmt" "time" ) func main() { go func() { fmt.Println("Hello from anonymous goroutine!") }() time.Sleep(1 * time.Second) fmt.Println("Hello from main!") } Output: Hello from anonymous goroutine! Hello from main! Synchronizing Goroutines In the above examples, time.Sleep is used to wait for the goroutine to finish. However, this is not a reliable method for synchronization. We'll explore better synchronization techniques, such as WaitGroups, later in this course. 3. Channels: Communication Between Goroutines What are Channels? Channels are Go's way of allowing goroutines to communicate with each other and synchronize their execution. They provide a safe way to send and receive data between goroutines, ensuring that data is exchanged without race conditions. Creating a Channel Use the make function to create a channel. ch := make(chan int) This creates a channel of type int. Sending and Receiving on Channels Send: Use the <- operator to send data into a channel. Receive: Use the <- operator to receive data from a channel. package main import ( "fmt" ) func main() { ch := make(chan string) // Sender goroutine go func() { ch <- "Hello from channel!" }() // Receiver msg := <-ch fmt.Println(msg) } Output: Hello from channel! Unidirectional Channels Channels can be bidirectional (default) or unidirectional (send-only or receive-only). Unidirectional channels are useful for enforcing communication directionality in your code. Send-Only: chan<- Type Receive-Only: <-chan Type func send(ch chan<- string, msg string) { ch <- msg } func receive(ch <-chan string) string { return <-ch } Buffered vs. Unbuffered Channels Unbuffered Channels: No capacity to hold values; send and receive operations block until the other side is ready. Buffered Channels: Have a capacity to hold a certain number of values; send operations block only when the buffer is full, and receive operations block only when the buffer is empty. We'll discuss this in detail in the next section. 4. Channel Operations Sending Data to a Channel Use the <- operator to send data. ch <- value Example: ch := make(chan int) go func() { ch <- 42 }() fmt.Println(<-ch) // Outputs: 42 Receiving Data from a Channel Use the <- operator to receive data. value := <-ch Example: ch := make(chan int) go func() { ch <- 42 }() value := <-ch fmt.Println(value) // Outputs: 42 Closing a Channel Use the close function to close a channel. This indicates that no more values will be sent on the channel. close(ch) Note: Only the sender should close a channel, never the receiver. Checking if a Channel is Closed When receiving from a closed channel, the operation returns the zero value of the channel's type and a boolean indicating if the channel is closed. value, ok := <-ch if !ok { fmt.Println("Channel closed!") } Example: ch := make(chan int) close(ch) value, ok := <-ch fmt.Println(value, ok) // Outputs: 0 false Range over Channels You can use a for range loop to receive values from a channel until it is closed. ch := make(chan int) go func() { for i := 0; i < 5; i++ { ch <- i } close(ch) }() for val := range ch { fmt.Println(val) } Output: 0 1 2 3 4 Select Statement The select statement allows a goroutine to wait on multiple communication operations. select { case msg1 := <-ch1: fmt.Println("Received", msg1) case ch2 <- msg2: fmt.Println("Sent", msg2) default: fmt.Println("No communication") } We'll explore select in detail in the later sections. 5. Buffered vs. Unbuffered Channels Unbuffered Channels Unbuffered channels have no capacity to store values. Both sender and receiver must be ready to perform the communication. Characteristics: Send operations block until a receiver is ready. Receive operations block until a sender sends a value. Use Cases: Synchronization between goroutines. Ensuring ordering of operations. Example: package main import ( "fmt" ) func main() { ch := make(chan int) // Unbuffered channel go func() { ch <- 10 // Blocks until receiver is ready fmt.Println("Sent 10") }() value := <-ch // Blocks until value is sent fmt.Println("Received", value) } Output: Received 10 Sent 10 Buffered Channels Buffered channels have a capacity to store a specified number of values without blocking. Characteristics: Send operations block only when the buffer is full. Receive operations block only when the buffer is empty. Allows decoupling between sender and receiver to some extent. Syntax: ch := make(chan Type, capacity) Example: package main import ( "fmt" ) func main() { ch := make(chan int, 2) // Buffered channel with capacity 2 ch <- 1 ch <- 2 fmt.Println("Sent two values") // The third send would block since the buffer is full // go func() { ch <- 3 }() fmt.Println(<-ch) fmt.Println(<-ch) } Output: Sent two values 1 2 When to Use Buffered Channels: When you need to allow some decoupling between sender and receiver. To improve performance by reducing the number of blocking operations. When implementing producer-consumer patterns where producers can produce ahead of consumers. Caveats: Overuse of buffered channels can lead to increased memory usage. Managing buffer sizes requires careful consideration to prevent deadlocks or memory leaks. 6. Channel Directionality Understanding Directionality Channels in Go can be bidirectional or unidirectional. Specifying directionality helps in enforcing communication patterns and improving code clarity. Bidirectional Channel: Can both send and receive. Send-Only Channel: Can only send data. Receive-Only Channel: Can only receive data. Declaring Channel Direction When passing channels to functions, you can specify their direction. Send-Only: func sendData(ch chan<- int, value int) { ch <- value } Receive-Only: func receiveData(ch <-chan int) int { return <-ch } Bidirectional (Default): func process(ch chan int) { ch <- 1 value := <-ch } Example: Using Unidirectional Channels package main import ( "fmt" ) func sendData(ch chan<- string, msg string) { ch <- msg } func receiveData(ch <-chan string) { msg := <-ch fmt.Println("Received:", msg) } func main() { ch := make(chan string) go sendData(ch, "Hello, Go!") receiveData(ch) } Output: Received: Hello, Go! Benefits of Using Unidirectional Channels Safety: Prevents accidental misuse by enforcing communication direction. Clarity: Makes the code easier to understand by specifying intent. Encapsulation: Helps in designing better APIs by controlling how channels are used. 7. The select Statement What is the select Statement? The select statement lets a goroutine wait on multiple communication operations (channel sends or receives). It's similar to the switch statement but specifically designed for channels. Basic Syntax select { case <-ch1: // Handle ch1 receive case ch2 <- value: // Handle ch2 send default: // Handle default case if no other case is ready } How select Works select blocks until at least one of its cases can proceed. If multiple cases are ready, one is chosen at random. The default case executes immediately if no other case is ready, preventing blocking. Example: Using select for Multiple Channels package main import ( "fmt" "time" ) func main() { ch1 := make(chan string) ch2 := make(chan string) // Goroutine sending to ch1 after 1 second go func() { time.Sleep(1 * time.Second) ch1 <- "Message from ch1" }() // Goroutine sending to ch2 after 2 seconds go func() { time.Sleep(2 * time.Second) ch2 <- "Message from ch2" }() for i := 0; i < 2; i++ { select { case msg1 := <-ch1: fmt.Println(msg1) case msg2 := <-ch2: fmt.Println(msg2) } } } Output: Message from ch1 Message from ch2 Using select with default Case The default case allows select to proceed without blocking if no other case is ready. select { case msg := <-ch: fmt.Println("Received:", msg) default: fmt.Println("No message received") } Example: package main import ( "fmt" ) func main() { ch := make(chan int) select { case msg := <-ch: fmt.Println("Received:", msg) default: fmt.Println("No message received") } } Output: No message received Using select with time.After You can use select with time.After to implement timeouts. select { case msg := <-ch: fmt.Println("Received:", msg) case <-time.After(2 * time.Second): fmt.Println("Timeout!") } Example: package main import ( "fmt" "time" ) func main() { ch := make(chan string) go func() { time.Sleep(3 * time.Second) ch <- "Delayed message" }() select { case msg := <-ch: fmt.Println("Received:", msg) case <-time.After(2 * time.Second): fmt.Println("Timeout!") } } Output: Timeout! Select with Multiple Cases select { case msg1 := <-ch1: fmt.Println("Received from ch1:", msg1) case msg2 := <-ch2: fmt.Println("Received from ch2:", msg2) case ch3 <- msg3: fmt.Println("Sent to ch3:", msg3) default: fmt.Println("No communication") } Note: Use select judiciously to avoid complexity and ensure readability. 8. Synchronization with WaitGroups What are WaitGroups? WaitGroups are used to wait for a collection of goroutines to finish executing. They provide a way to synchronize concurrent operations, ensuring that the main function waits until all goroutines have completed. Using sync.WaitGroup The sync.WaitGroup type from the sync package provides the necessary functionality. Basic Operations Add(delta int): Adds the number of goroutines to wait for. Done(): Decrements the WaitGroup counter by one. Wait(): Blocks until the WaitGroup counter is zero. Example: Waiting for Goroutines to Finish package main import ( "fmt" "sync" "time" ) func worker(id int, wg *sync.WaitGroup) { defer wg.Done() fmt.Printf("Worker %d starting\n", id) time.Sleep(time.Second) fmt.Printf("Worker %d done\n", id) } func main() { var wg sync.WaitGroup for i := 1; i <= 3; i++ { wg.Add(1) go worker(i, &wg) } wg.Wait() fmt.Println("All workers done") } Output: Worker 1 starting Worker 2 starting Worker 3 starting Worker 1 done Worker 2 done Worker 3 done All workers done Common Patterns Adding to WaitGroup Before Starting Goroutines Ensure you call wg.Add(1) before starting the goroutine to prevent race conditions. wg.Add(1) go func() { defer wg.Done() // Do work }() Deferring wg.Done() Use defer wg.Done() at the beginning of the goroutine to ensure it is called even if the goroutine panics. Waiting in the Main Goroutine The main goroutine should call wg.Wait() to block until all other goroutines have called wg.Done(). Example: Concurrent Downloads with WaitGroup package main import ( "fmt" "math/rand" "sync" "time" ) func downloadFile(file string, wg *sync.WaitGroup) { defer wg.Done() fmt.Printf("Downloading %s...\n", file) time.Sleep(time.Duration(rand.Intn(3)) * time.Second) fmt.Printf("Finished downloading %s\n", file) } func main() { rand.Seed(time.Now().UnixNano()) files := []string{"file1.txt", "file2.jpg", "file3.pdf"} var wg sync.WaitGroup for _, file := range files { wg.Add(1) go downloadFile(file, &wg) } wg.Wait() fmt.Println("All downloads completed.") } Sample Output: Downloading file1.txt... Downloading file2.jpg... Downloading file3.pdf... Finished downloading file2.jpg Finished downloading file1.txt Finished downloading file3.pdf All downloads completed. 9. Avoiding Common Concurrency Pitfalls Concurrency introduces complexity, and it's easy to introduce bugs if not handled carefully. Here are some common pitfalls and how to avoid them. 1. Race Conditions Race conditions occur when multiple goroutines access shared data concurrently without proper synchronization. Solution: Use channels to communicate and synchronize access. Use mutexes (sync.Mutex) to protect shared data. Use atomic operations (sync/atomic) for simple cases. Example of Race Condition: package main import ( "fmt" "sync" ) func main() { var counter int var wg sync.WaitGroup for i := 0; i < 1000; i++ { wg.Add(1) go func() { counter++ wg.Done() }() } wg.Wait() fmt.Println("Counter:", counter) // May not be 1000 } Solution with Mutex: package main import ( "fmt" "sync" ) func main() { var counter int var mu sync.Mutex var wg sync.WaitGroup for i := 0; i < 1000; i++ { wg.Add(1) go func() { mu.Lock() counter++ mu.Unlock() wg.Done() }() } wg.Wait() fmt.Println("Counter:", counter) // Always 1000 } 2. Deadlocks A deadlock occurs when goroutines are waiting indefinitely for each other, preventing progress. Common Causes: Improper use of channels (e.g., both sender and receiver waiting). Not closing channels when expected. Circular dependencies. Example of Deadlock: package main func main() { ch := make(chan int) ch <- 1 // Blocks forever since no receiver } Solution: Ensure that for every send, there is a corresponding receive, and vice versa. package main import "fmt" func main() { ch := make(chan int) go func() { ch <- 1 }() fmt.Println(<-ch) } 3. Goroutine Leaks A goroutine leak happens when goroutines are left running without a way to terminate, often due to blocking operations. Solution: Use select with done channels or context cancellation to signal goroutines to exit. Ensure all goroutines have a clear exit path. Example of Goroutine Leak: package main func main() { ch := make(chan int) go func() { for { <-ch // Never exits } }() } Solution with done Channel: package main import ( "fmt" ) func worker(ch <-chan int, done <-chan bool) { for { select { case val := <-ch: fmt.Println("Received:", val) case <-done: fmt.Println("Exiting goroutine") return } } } func main() { ch := make(chan int) done := make(chan bool) go worker(ch, done) ch <- 1 ch <- 2 done <- true } Output: Received: 1 Received: 2 Exiting goroutine 4. Improper Channel Closure Closing a channel incorrectly can lead to panics or unexpected behavior. Rules for Closing Channels: Only the sender should close a channel. Never close a channel from the receiver side. Do not close a channel multiple times. Receivers should handle closed channels gracefully. Example of Improper Closure: package main func main() { ch := make(chan int) close(ch) close(ch) // Panic: close of closed channel } Proper Closure: package main import "fmt" func main() { ch := make(chan int) go func() { ch <- 1 ch <- 2 close(ch) }() for val := range ch { fmt.Println(val) } } Output: 1 2 10. Concurrency Patterns and Best Practices 1. Worker Pool Pattern A worker pool limits the number of concurrent goroutines performing a task, improving resource management and preventing overload. Example: package main import ( "fmt" "sync" "time" ) func worker(id int, jobs <-chan int, wg *sync.WaitGroup) { defer wg.Done() for job := range jobs { fmt.Printf("Worker %d processing job %d\n", id, job) time.Sleep(time.Second) } } func main() { const numWorkers = 3 jobs := make(chan int, 5) var wg sync.WaitGroup for w := 1; w <= numWorkers; w++ { wg.Add(1) go worker(w, jobs, &wg) } for j := 1; j <= 5; j++ { jobs <- j } close(jobs) wg.Wait() fmt.Println("All jobs processed.") } Output: Worker 1 processing job 1 Worker 2 processing job 2 Worker 3 processing job 3 Worker 1 processing job 4 Worker 2 processing job 5 All jobs processed. 2. Pipeline Pattern A pipeline allows data to flow through a series of stages, each processing the data and passing it to the next stage. Example: package main import ( "fmt" ) func generator(nums ...int) <-chan int { out := make(chan int) go func() { for _, n := range nums { out <- n } close(out) }() return out } func square(in <-chan int) <-chan int { out := make(chan int) go func() { for n := range in { out <- n * n } close(out) }() return out } func main() { nums := generator(2, 3, 4, 5) squares := square(nums) for sq := range squares { fmt.Println(sq) } } Output: 4 9 16 25 3. Fan-In and Fan-Out Fan-Out: Distributing work across multiple goroutines. Fan-In: Merging multiple channels into one. Example: package main import ( "fmt" "sync" ) func worker(id int, jobs <-chan int, results chan<- int, wg *sync.WaitGroup) { defer wg.Done() for job := range jobs { fmt.Printf("Worker %d processing job %d\n", id, job) results <- job * 2 } } func main() { jobs := make(chan int, 5) results := make(chan int, 5) var wg sync.WaitGroup // Start 3 workers for w := 1; w <= 3; w++ { wg.Add(1) go worker(w, jobs, results, &wg) } // Send jobs for j := 1; j <= 5; j++ { jobs <- j } close(jobs) // Wait for workers to finish go func() { wg.Wait() close(results) }() // Collect results for res := range results { fmt.Println("Result:", res) } } Output: Worker 1 processing job 1 Worker 2 processing job 2 Worker 3 processing job 3 Worker 1 processing job 4 Worker 2 processing job 5 Result: 2 Result: 4 Result: 6 Result: 8 Result: 10 4. Rate Limiting Control the rate at which events are processed using channels and time.Ticker. Example: package main import ( "fmt" "time" ) func main() { jobs := make(chan int) done := make(chan bool) // Rate limiter: one job per second limiter := time.Tick(1 * time.Second) go func() { for j := range jobs { <-limiter fmt.Println("Processing job", j) } done <- true }() for j := 1; j <= 5; j++ { jobs <- j } close(jobs) <-done } Output: Processing job 1 Processing job 2 Processing job 3 Processing job 4 Processing job 5 Note: Each job is processed at a rate of one per second. 5. Fan-Out/Fan-In with Multiple Stages Example: package main import ( "fmt" "sync" ) func generator(nums ...int) <-chan int { out := make(chan int) go func() { for _, n := range nums { out <- n } close(out) }() return out } func square(in <-chan int) <-chan int { out := make(chan int) go func() { for n := range in { out <- n * n } close(out) }() return out } func cube(in <-chan int) <-chan int { out := make(chan int) go func() { for n := range in { out <- n * n * n } close(out) }() return out } func merge(cs ...<-chan int) <-chan int { var wg sync.WaitGroup out := make(chan int) // Start an output goroutine for each input channel in cs. // Each goroutine copies values from its input channel to out until the channel is closed. for _, c := range cs { wg.Add(1) go func(c <-chan int) { defer wg.Done() for n := range c { out <- n } }(c) } // Start a goroutine to close out once all the output goroutines are done. go func() { wg.Wait() close(out) }() return out } func main() { nums := generator(2, 3, 4) squares := square(nums) cubes := cube(nums) for result := range merge(squares, cubes) { fmt.Println(result) } } Output: 4 8 9 27 16 64 11. Advanced Topics 11.1. Context Package The context package in Go provides a way to carry deadlines, cancellation signals, and other request-scoped values across API boundaries and between processes. Use Cases: Timeouts and cancellations for goroutines. Passing request-scoped data. Managing goroutine lifecycles. Basic Usage: package main import ( "context" "fmt" "time" ) func worker(ctx context.Context) { for { select { case <-ctx.Done(): fmt.Println("Worker stopping:", ctx.Err()) return default: fmt.Println("Worker working...") time.Sleep(500 * time.Millisecond) } } } func main() { ctx, cancel := context.WithCancel(context.Background()) go worker(ctx) time.Sleep(2 * time.Second) cancel() time.Sleep(1 * time.Second) fmt.Println("Main done") } Output: Worker working... Worker working... Worker working... Worker working... Worker stopping: context canceled Main done Common Functions: context.Background(): Returns an empty context. context.TODO(): Used when unsure which context to use. context.WithCancel(parent): Returns a copy of the parent context with a cancellation function. context.WithTimeout(parent, timeout): Returns a copy of the parent context with a deadline. context.WithDeadline(parent, deadline): Returns a copy of the parent context with a specific deadline. Example with Timeout: package main import ( "context" "fmt" "time" ) func worker(ctx context.Context) { for { select { case <-ctx.Done(): fmt.Println("Worker stopping:", ctx.Err()) return default: fmt.Println("Worker working...") time.Sleep(500 * time.Millisecond) } } } func main() { ctx, cancel := context.WithTimeout(context.Background(), 2*time.Second) defer cancel() go worker(ctx) <-ctx.Done() fmt.Println("Main done:", ctx.Err()) } Output: Worker working... Worker working... Worker working... Worker stopping: context deadline exceeded Main done: context deadline exceeded 11.2. Pipelines A pipeline is a series of connected stages, each processing data and passing it to the next stage. Pipelines are useful for processing streams of data efficiently. Example: package main import ( "fmt" "strings" ) func generator(nums ...int) <-chan int { out := make(chan int) go func() { for _, n := range nums { out <- n } close(out) }() return out } func square(in <-chan int) <-chan int { out := make(chan int) go func() { for n := range in { out <- n * n } close(out) }() return out } func toString(in <-chan int) <-chan string { out := make(chan string) go func() { for n := range in { out <- fmt.Sprintf("Number: %d", n) } close(out) }() return out } func main() { nums := generator(2, 3, 4) squares := square(nums) stringsChan := toString(squares) for s := range stringsChan { fmt.Println(s) } } Output: Number: 4 Number: 9 Number: 16 11.3. Fan-In/Fan-Out Fan-Out: Distributing work across multiple goroutines. Fan-In: Merging results from multiple goroutines into a single channel. Example: package main import ( "fmt" "sync" "time" ) func worker(id int, jobs <-chan int, results chan<- int, wg *sync.WaitGroup) { defer wg.Done() for job := range jobs { fmt.Printf("Worker %d processing job %d\n", id, job) time.Sleep(time.Millisecond * 500) results <- job * 2 } } func fanIn(cs ...<-chan int) <-chan int { var wg sync.WaitGroup out := make(chan int) output := func(c <-chan int) { defer wg.Done() for n := range c { out <- n } } wg.Add(len(cs)) for _, c := range cs { go output(c) } go func() { wg.Wait() close(out) }() return out } func main() { jobs := make(chan int, 5) results := make(chan int, 5) var wg sync.WaitGroup // Start 2 workers for w := 1; w <= 2; w++ { wg.Add(1) go worker(w, jobs, results, &wg) } // Send jobs for j := 1; j <= 4; j++ { jobs <- j } close(jobs) // Collect results go func() { wg.Wait() close(results) }() for res := range results { fmt.Println("Result:", res) } } Output: Worker 1 processing job 1 Worker 2 processing job 2 Worker 1 processing job 3 Worker 2 processing job 4 Result: 2 Result: 4 Result: 6 Result: 8 11.4. Worker Pools A worker pool is a collection of goroutines that process tasks from a shared channel. It limits the number of concurrent tasks, improving resource management. Example: package main import ( "fmt" "sync" "time" ) func worker(id int, jobs <-chan int, results chan<- int, wg *sync.WaitGroup) { defer wg.Done() for job := range jobs { fmt.Printf("Worker %d processing job %d\n", id, job) time.Sleep(time.Millisecond * 300) results <- job * 2 } } func main() { const numWorkers = 3 jobs := make(chan int, 5) results := make(chan int, 5) var wg sync.WaitGroup // Start worker pool for w := 1; w <= numWorkers; w++ { wg.Add(1) go worker(w, jobs, results, &wg) } // Send jobs for j := 1; j <= 5; j++ { jobs <- j } close(jobs) // Collect results go func() { wg.Wait() close(results) }() for res := range results { fmt.Println("Result:", res) } } Output: Worker 1 processing job 1 Worker 2 processing job 2 Worker 3 processing job 3 Worker 1 processing job 4 Worker 2 processing job 5 Result: 2 Result: 4 Result: 6 Result: 8 Result: 10 12. Practical Examples and Projects Example 1: Concurrent Web Scraper A web scraper that fetches URLs concurrently using goroutines and channels. Requirements: Fetch multiple URLs concurrently. Limit the number of concurrent fetches. Collect and display the results. Implementation: package main import ( "fmt" "io/ioutil" "net/http" "sync" ) func fetchURL(url string, wg *sync.WaitGroup, ch chan<- string) { defer wg.Done() resp, err := http.Get(url) if err != nil { ch <- fmt.Sprintf("Error fetching %s: %v", url, err) return } defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { ch <- fmt.Sprintf("Error reading %s: %v", url, err) return } ch <- fmt.Sprintf("Fetched %s: %d bytes", url, len(body)) } func main() { urls := []string{ "https://www.google.com", "https://www.github.com", "https://www.golang.org", "https://www.stackoverflow.com", } var wg sync.WaitGroup ch := make(chan string, len(urls)) for _, url := range urls { wg.Add(1) go fetchURL(url, &wg, ch) } wg.Wait() close(ch) for msg := range ch { fmt.Println(msg) } } Sample Output: Fetched https://www.google.com: 12500 bytes Fetched https://www.github.com: 10500 bytes Fetched https://www.golang.org: 9500 bytes Fetched https://www.stackoverflow.com: 11500 bytes Example 2: Real-Time Chat Application A simple real-time chat application using goroutines and channels. Features: Multiple clients can send and receive messages. Messages are broadcasted to all connected clients. Implementation: Due to space constraints, a simplified version is presented. package main import ( "bufio" "fmt" "net" "sync" ) var ( clients = make(map[net.Conn]bool) broadcast = make(chan string) mu sync.Mutex ) func handleConnection(conn net.Conn) { defer conn.Close() mu.Lock() clients[conn] = true mu.Unlock() reader := bufio.NewReader(conn) for { msg, err := reader.ReadString('\n') if err != nil { break } broadcast <- msg } mu.Lock() delete(clients, conn) mu.Unlock() } func broadcaster() { for { msg := <-broadcast mu.Lock() for conn := range clients { fmt.Fprint(conn, msg) } mu.Unlock() } } func main() { listener, err := net.Listen("tcp", ":9000") if err != nil { fmt.Println("Error starting server:", err) return } defer listener.Close() fmt.Println("Chat server started on :9000") go broadcaster() for { conn, err := listener.Accept() if err != nil { fmt.Println("Error accepting connection:", err) continue } go handleConnection(conn) } } Usage: Run the server. Connect multiple clients using telnet or nc: telnet localhost 9000 Type messages in one client and see them broadcasted to all connected clients. Example 3: Parallel Data Processing with Pipelines A pipeline that processes data in stages: generation, processing, and consumption. Implementation: package main import ( "fmt" "math/rand" "time" ) func generator(count int) <-chan int { out := make(chan int) go func() { for i := 0; i < count; i++ { out <- rand.Intn(100) time.Sleep(time.Millisecond * 100) } close(out) }() return out } func processor(in <-chan int) <-chan int { out := make(chan int) go func() { for num := range in { out <- num * num time.Sleep(time.Millisecond * 200) } close(out) }() return out } func consumer(in <-chan int, done chan<- bool) { for num := range in { fmt.Println("Processed number:", num) } done <- true } func main() { rand.Seed(time.Now().UnixNano()) done := make(chan bool) nums := generator(5) squares := processor(nums) go consumer(squares, done) <-done fmt.Println("All numbers processed.") } Sample Output: Processed number: 1024 Processed number: 576 Processed number: 841 Processed number: 144 Processed number: 900 All numbers processed. 13. Conclusion and Next Steps Congratulations! You've completed the comprehensive course on Goroutines and Channels in Go. Here's a summary of what you've learned: Goroutines: Lightweight threads for concurrent execution. Channels: Safe communication between goroutines. Channel Operations: Sending, receiving, closing, and ranging over channels. Buffered vs. Unbuffered Channels: Understanding different channel capacities. Channel Directionality: Enforcing communication patterns. Select Statement: Handling multiple channel operations. WaitGroups: Synchronizing goroutines. Concurrency Pitfalls: Avoiding race conditions, deadlocks, and goroutine leaks. Concurrency Patterns: Implementing worker pools, pipelines, fan-in/fan-out, and more. Advanced Topics: Using the context package for cancellation and timeouts. Next Steps To further solidify your understanding and expertise in Go concurrency: Build Real-World Projects: Apply the concepts by building applications such as web servers, concurrent data processors, or real-time systems. Explore Go's Standard Library: Many packages in Go's standard library make extensive use of concurrency. Studying these can provide deeper insights. Performance Optimization: Learn how to profile and optimize concurrent Go programs for better performance. Advanced Synchronization: Dive deeper into synchronization primitives like mutexes, condition variables, and atomic operations. Understand Go's Scheduler: Learn how Go's runtime scheduler manages goroutines and threads for better optimization. Stay Updated: Go continues to evolve. Keep up with the latest features and best practices by following official Go blogs, forums, and community discussions. Recommended Resources Books: The Go Programming Language by Alan A. A. Donovan & Brian W. Kernighan Concurrency in Go by Katherine Cox-Buday Online Tutorials: Go by Example: Goroutines Go by Example: Channels Official Documentation: Go Concurrency Patterns Go Context Package Courses: Udemy: Learn How To Code: Google's Go Programming Language Coursera: Programming with Google Go Thank you for embarking on this journey to master Go's concurrency model! With practice and exploration, you'll be well-equipped to build efficient, concurrent applications in Go. Happy Coding!
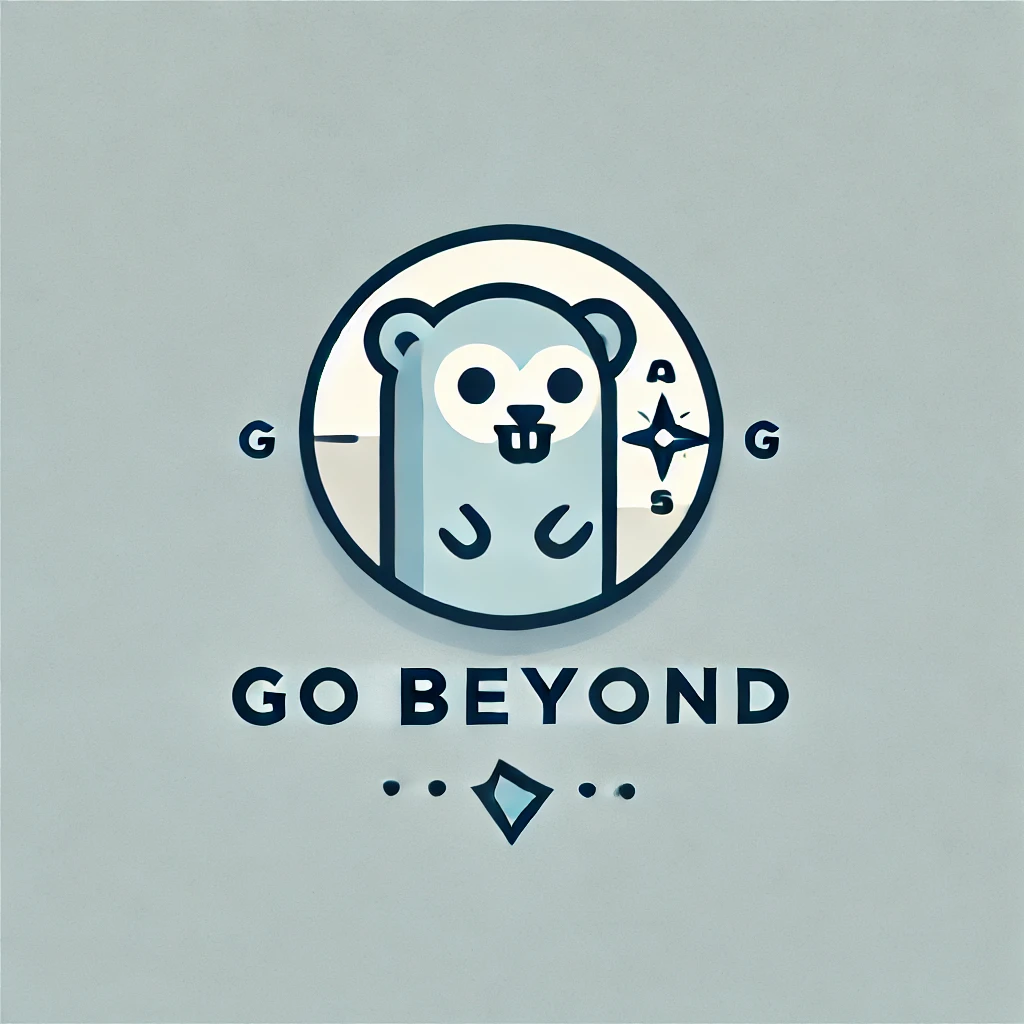
2024 Beginner's Guide to Learning Go (Golang)

A Journey into Modern Programming Excellence Welcome, fellow developers and coding enthusiasts! Whether you're a veteran programmer exploring new horizons or taking your first steps into the programming world, you're about to embark on an exciting journey with one of the most transformative programming languages of our time. Table of Contents Introduction: Why Go Matters in 2024 The Story Behind Go The Go Revolution: Why It Was Created Getting Started with Go Your First Go Adventure Go's Evolution: From Birth to Maturity The Go Developer's Mindset Learning Resources and Community What's Next in Your Go Journey Introduction: Why Go Matters in 2024 {#introduction} In today's tech landscape, where complexity often reigns supreme, Go stands as a beacon of clarity and efficiency. But what makes Go particularly relevant in 2024? The Modern Developer's Swiss Army Knife Cloud-Native Development: With the explosion of cloud computing and microservices, Go has become the de facto language for cloud-native applications AI/ML Infrastructure: Companies like OpenAI use Go for their infrastructure components DevOps & SRE: Major tools like Kubernetes, Docker, and Terraform are written in Go Web Services: From high-performance APIs to real-time web applications Key Statistics (2024) Over 2.5 million developers worldwide use Go Consistently ranks in the top 10 most loved languages on Stack Overflow Average Go developer salary: $140,000+ (US) 76% of developers report improved productivity after switching to Go The Story Behind Go {#history} The Perfect Storm at Google Picture this: It's 2007, and Google's engineers are drowning in a sea of complexity. Build times are measured in coffee breaks, code bases are becoming unmanageable, and multicore processors are becoming the norm, but programming languages haven't caught up. The Dream Team Three extraordinary minds came together: Robert Griesemer: The language design expert who helped create the V8 JavaScript engine Rob Pike: The Unix veteran and UTF-8 co-creator Ken Thompson: The legendary Bell Labs researcher who gave us Unix and C Their mission? Create a language that would: Compile at the speed of thought Make concurrent programming easy and safe Be readable enough that you could understand someone else's code without a manual Scale from small scripts to massive systems Why Go Was Created {#why-go} The Problems Go Solves The Complexity Crisis C++ and Java's overwhelming feature sets Python's performance limitations Lack of built-in concurrency support in mainstream languages Modern Computing Challenges Multicore processors being underutilized Distributed systems becoming the norm Build times affecting developer productivity Dependencies becoming unmanageable Go's Solutions Simplicity by Design // Example of Go's simplicity type User struct { Name string Age int } func (u User) Greet() string { return fmt.Sprintf("Hello, I'm %s!", u.Name) } Built-in Concurrency // Example of concurrent programming in Go func processItems(items []string) { for _, item := range items { go func(i string) { // Process each item concurrently fmt.Println("Processing:", i) }(item) } } Getting Started with Go {#installation} Modern Installation Methods (2024) Official Installation # Windows (using winget) winget install GoLang.Go # macOS (using Homebrew) brew install go # Linux (using apt) sudo apt install golang-go Using Docker FROM golang:1.22 WORKDIR /app Environment Setup # Create your Go workspace mkdir -p ~/go/{bin,src,pkg} # Add to your profile (.bashrc, .zshrc, etc.) export GOPATH=$HOME/go export PATH=$PATH:$GOPATH/bin Your First Go Adventure {#first-program} The Modern "Hello, World!" package main import ( "fmt" "time" ) func main() { name := "Gopher" currentTime := time.Now().Format("15:04:05") fmt.Printf("Hello, %s! The time is %s\n", name, currentTime) } Understanding the Code Package Declaration: Every Go file starts with package main Imports: Standard library packages are imported using their path Main Function: The entry point of every Go program Variable Declaration: Using the := shorthand for declaration and assignment String Formatting: Using Printf for formatted output Go's Evolution: From Birth to Maturity {#evolution} Recent Milestones (2022-2024) Go 1.18: Introduction of Generics Go 1.19: Performance improvements in the garbage collector Go 1.20: Enhanced error handling and fuzzing Go 1.21: Built-in slices package and faster compilation Go 1.22: Enhanced HTTP routing and improved tooling Go 1.23: (Expected) Further performance optimizations Modern Features Showcase // Generics Example (Go 1.18+) func Min[T constraints.Ordered](x, y T) T { if x < y { return x } return y } // Error Handling (Modern Approach) if err := doSomething(); err != nil { err = fmt.Errorf("failed to do something: %w", err) return err } The Go Developer's Mindset {#mindset} Core Principles for 2024 Embrace Simplicity Clear is better than clever Explicit is better than implicit Small functions and packages Think Concurrently Goroutines are cheap, use them Channels for communication Share memory by communicating Performance Matters Profile before optimizing Use benchmarks Understand the garbage collector Best Practices // Good Practice: Use meaningful names func ProcessUserData(user User) error { // vs "func process(u User)" // Good Practice: Error handling if err != nil { return fmt.Errorf("failed to process user %s: %w", user.ID, err) } // Good Practice: Structured logging log.WithFields(log.Fields{ "user_id": user.ID, "action": "process", }).Info("Processing user data") Learning Resources and Community {#resources} Modern Learning Paths Interactive Learning Go Playground (Updated 2024) Tour of Go Exercism's Go Track Books & Documentation "Learning Go" (2024 Edition) "Go Programming Language" (Classic) Official Go Blog Community Resources Go Discord Server r/golang Local Go Meetups Recommended Project Ideas Beginner CLI tool for file management Simple REST API Task manager Intermediate Chat application using WebSockets Microservice with gRPC Custom database driver Advanced Kubernetes operator Distributed cache system High-performance web server What's Next in Your Go Journey {#conclusion} Immediate Next Steps Set up your Go development environment Complete the Tour of Go Build your first command-line tool Join the Go community on Discord or Reddit Looking Ahead The Go ecosystem is continuously evolving, with exciting developments in: AI/ML tooling WebAssembly support Cloud-native development Performance optimizations Final Thoughts Go's journey from a solution to Google's problems to a mainstream programming language is a testament to its design principles. As you begin your Go journey, remember that simplicity is your friend, and the Go community is here to help. Stay tuned for our next article in the series, where we'll dive deep into Go's type system and explore how to write idiomatic Go code! Did you find this guide helpful? Share it with your network and let us know your thoughts in the comments below! Don't forget to follow for more Go content.
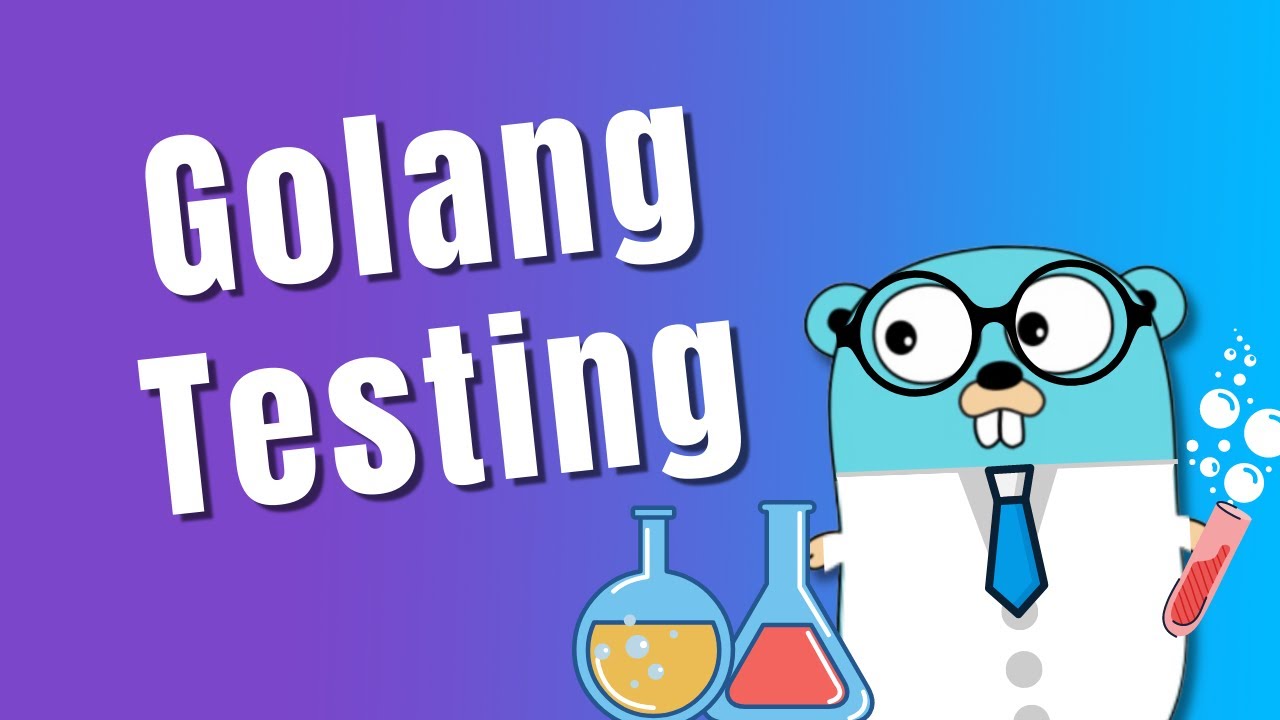
Ultimate Guide to Go Testing: Basic to Expert Techniques

Welcome to your journey towards mastering testing in Go! Testing is a crucial aspect of software development that ensures your code works as intended and remains maintainable over time. In this comprehensive guide, we'll start from the basics and progress to advanced testing techniques and patterns used in Go (Golang). Let's dive in! Table of Contents Introduction to Testing in Go Basic Testing in Go Table-Driven Tests Subtests and Sub-benchmarks Benchmark Testing Coverage Analysis Mocking and Dependency Injection Test Suites with testify Behavior-Driven Development (BDD) Advanced Testing Techniques Testing Patterns Best Practices Continuous Integration and Testing Tips for Mastery Introduction to Testing in Go Importance of Testing Reliability: Ensures your code behaves as expected. Maintainability: Facilitates refactoring and adding new features without breaking existing functionality. Documentation: Tests serve as live documentation for your codebase. Confidence: Provides assurance when deploying to production. Overview of Testing in Go Go provides a built-in testing package that makes writing tests straightforward. Testing in Go is: Simple: Minimal boilerplate code required. Integrated: The go test command is part of the Go toolchain. Extensible: Supports writing benchmarks and examples. Basic Testing in Go The testing Package The testing package provides support for automated testing of Go packages. It defines: Types: testing.T for tests, testing.B for benchmarks. Functions: Methods to report test failures. Writing Your First Test Function Let's start with a simple function to test: // math.go package math func Add(a, b int) int { return a + b } Now, write a test: // math_test.go package math import "testing" func TestAdd(t *testing.T) { sum := Add(2, 3) if sum != 5 { t.Errorf("Expected sum 5, but got %d", sum) } } Running Tests Run all tests in the current package: go test Verbose output: go test -v Table-Driven Tests What Are Table-Driven Tests? A testing style where test inputs and expected outputs are defined in a table (slice), and a loop iterates over them. Why Use Them? Scalability: Easily add new test cases. Readability: Organizes tests neatly. Maintainability: Centralizes test data. Example func TestAddTableDriven(t *testing.T) { tests := []struct { name string a, b int expected int }{ {"Positive numbers", 2, 3, 5}, {"Zero", 0, 0, 0}, {"Negative numbers", -1, -1, -2}, } for _, tt := range tests { t.Run(tt.name, func(t *testing.T) { result := Add(tt.a, tt.b) if result != tt.expected { t.Errorf("Add(%d, %d) = %d; want %d", tt.a, tt.b, result, tt.expected) } }) } } Subtests and Sub-benchmarks Using t.Run() Allows grouping related tests and running them as subtests. func TestFeatures(t *testing.T) { t.Run("FeatureA", func(t *testing.T) { // Test code for FeatureA }) t.Run("FeatureB", func(t *testing.T) { // Test code for FeatureB }) } Organizing Tests Isolation: Subtests run independently. Parallelism: Use t.Parallel() inside subtests for concurrent execution. Benchmark Testing The testing.B Type Used for writing benchmarks to measure performance. Writing Benchmarks func BenchmarkAdd(b *testing.B) { for i := 0; i < b.N; i++ { Add(1, 2) } } Example Run the benchmark: go test -bench=. Coverage Analysis go test -cover Measures code coverage of your tests. go test -cover Interpreting Coverage Reports Coverage Percentage: Indicates how much of your code is tested. HTML Report: go test -coverprofile=coverage.out go tool cover -html=coverage.out Mocking and Dependency Injection Why Mocking Is Important Isolation: Test components independently. Control: Simulate different scenarios. Speed: Avoid slow operations (e.g., network calls). How to Mock in Go Use interfaces to define dependencies. Implement mock versions for testing. Using Interfaces for Dependency Injection type Fetcher interface { Fetch(url string) (string, error) } func GetTitle(f Fetcher, url string) (string, error) { return f.Fetch(url) } Example type MockFetcher struct{} func (m MockFetcher) Fetch(url string) (string, error) { return "Mock Title", nil } func TestGetTitle(t *testing.T) { mock := MockFetcher{} title, err := GetTitle(mock, "http://example.com") if err != nil || title != "Mock Title" { t.Fail() } } Test Suites with testify Using the testify Package testify provides advanced assertions and suite management. Advantages Enhanced Assertions: Readable failure messages. Test Suites: Setup and teardown methods. Examples import ( "testing" "github.com/stretchr/testify/assert" ) func TestSomething(t *testing.T) { assert.Equal(t, expected, actual, "they should be equal") } Behavior-Driven Development (BDD) Using GoConvey and Ginkgo GoConvey: Offers live reload and web UI. Ginkgo: BDD-style testing framework. Examples with Ginkgo import ( . "github.com/onsi/ginkgo" . "github.com/onsi/gomega" ) var _ = Describe("Add", func() { It("should add two numbers", func() { Expect(Add(1, 2)).To(Equal(3)) }) }) Advanced Testing Techniques Testing with Context Pass context.Context to manage timeouts and cancellations. Useful for testing functions that depend on context. Testing for Concurrency Race Conditions: Use go test -race to detect race conditions. Using the Race Detector go test -race Fuzz Testing Introduced in Go 1.18. Fuzzing: Automatic test generation with random inputs. func FuzzAdd(f *testing.F) { f.Add(1, 2) f.Fuzz(func(t *testing.T, a int, b int) { Add(a, b) }) } Testing Patterns Arrange-Act-Assert (AAA) Arrange: Set up the test data and environment. Act: Execute the code under test. Assert: Verify the result. Given-When-Then Similar to AAA but emphasizes the scenario. Why Patterns Are Used Clarity: Makes tests easier to read and understand. Consistency: Standardizes the structure of tests. Examples func TestCalculateDiscount(t *testing.T) { // Arrange price := 100.0 discount := 0.1 // Act finalPrice := CalculateDiscount(price, discount) // Assert if finalPrice != 90.0 { t.Errorf("Expected final price 90.0, got %f", finalPrice) } } Best Practices Organizing Tests Keep test files in the same package. Name test files with _test.go suffix. Naming Conventions Test functions start with Test. Use descriptive test names. Writing Maintainable Tests Avoid logic in tests. Keep tests independent. Test Failure Messages Provide clear and informative messages. Avoiding Common Pitfalls Don't rely on test execution order. Clean up resources in tests. Continuous Integration and Testing Using CI/CD Pipelines Automate testing with CI tools: Travis CI CircleCI GitHub Actions Integrating with GitHub Actions name: Go on: push: branches: [ main ] jobs: build: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - name: Set up Go uses: actions/setup-go@v2 with: go-version: 1.17 - name: Test run: go test -v ./... Tips for Mastery Read Standard Library Tests Learn from Go's standard library test code. Keep Learning and Practicing Experiment with different testing tools. Stay updated with the Go community. Code Reviews and Pair Programming Review others' tests. Collaborate to improve testing skills. Congratulations! You've covered a comprehensive journey from the basics of testing in Go to advanced techniques and patterns. Remember, mastering testing is an ongoing process that involves continuous learning and practice. Happy testing!
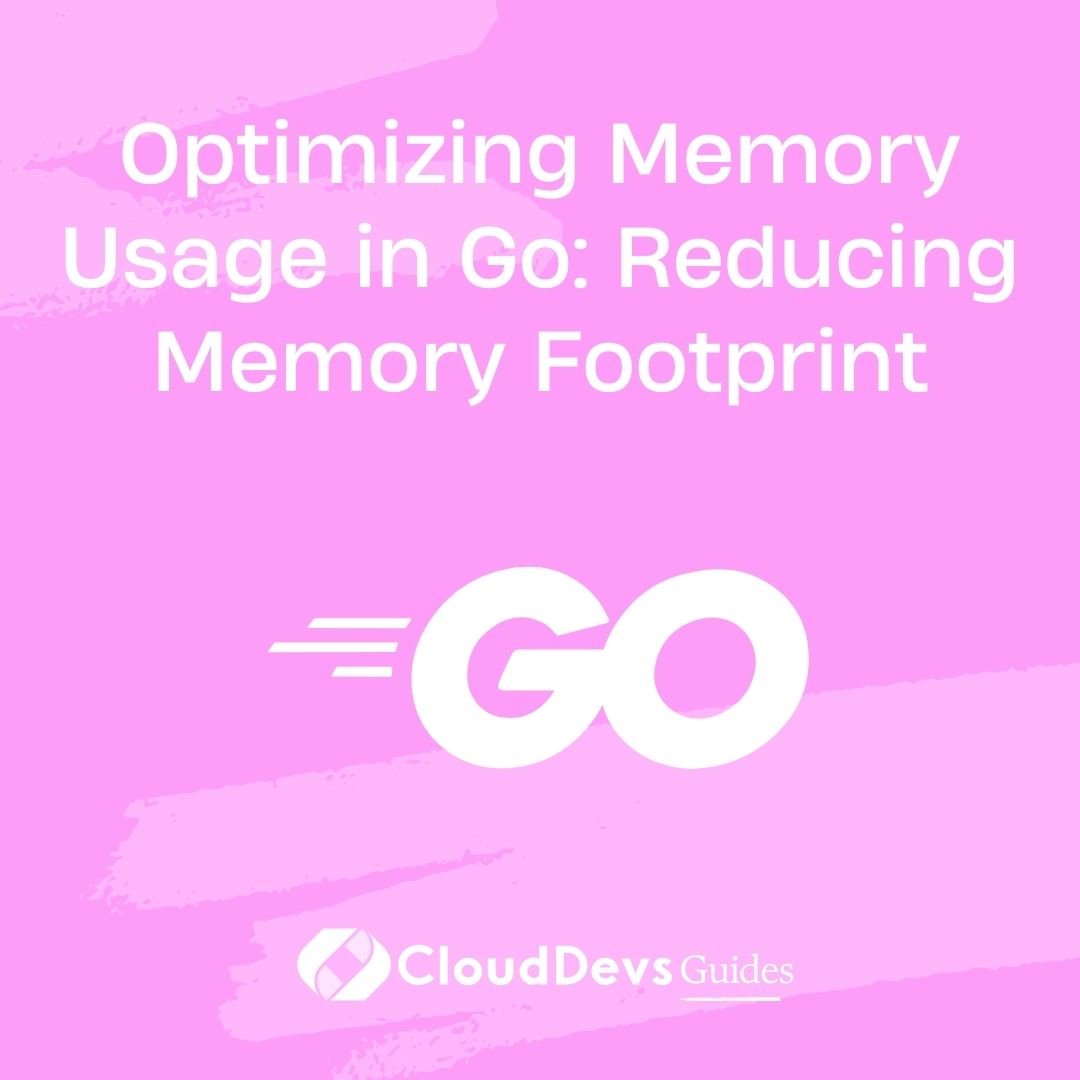
Understanding Profile-Guided Optimization (PGO) in Go

Profile-Guided Optimization (PGO), also known as Feedback-Directed Optimization (FDO), is a powerful compiler technique that enhances the performance of applications by using real-world data to inform optimization decisions during the build process. Starting from Go version 1.20, the Go compiler supports PGO to further optimize Go programs. What is PGO? PGO involves the following steps: Collecting Profiling Data: Run your application to gather profiling information that reflects typical usage patterns. This data is usually collected from production environments to ensure it is representative. Feeding Data Back to the Compiler: Use the collected profile during the next build of your application. The compiler utilizes this information to optimize the code more effectively. Optimizing Based on Actual Usage: The compiler may choose to inline frequently called functions, unroll loops, or make other optimizations that are beneficial based on the profiling data. Benefits of Using PGO in Go Performance Improvements: Benchmarks have shown that using PGO can improve the performance of Go applications by approximately 2-14%. Smarter Optimizations: The compiler makes more informed decisions, leading to better-optimized binaries that perform well under real-world conditions. Future Enhancements: As Go continues to evolve, more optimizations will be introduced, potentially increasing the benefits of PGO. How to Collect Profiles To use PGO effectively, you need to collect CPU profiles that represent your application's typical workload: Use Go's Profiling Tools: Employ the runtime/pprof or net/http/pprof packages to collect CPU profiles. These profiles record which parts of your code are most active during execution. Collect from Production Environments: Profiles should be gathered from production or environments that closely mimic production to ensure they are representative. Unrepresentative profiles may not lead to performance gains and could even overlook critical optimizations. Merge Multiple Profiles if Necessary: If your application has varying workloads, consider collecting multiple profiles and merging them using the pprof tool: go tool pprof -proto profile1.pprof profile2.pprof > merged.pprof Building with PGO After collecting the profiles, you can build your Go application using PGO: Save the Profile Appropriately: Place the profile file (e.g., default.pgo) in the main package directory of your application. Automatic Detection: By default, go build will detect default.pgo and use it for PGO without additional flags. Using the -pgo Flag: If you have profiles with different names or locations, specify the profile using the -pgo flag: go build -pgo=/path/to/your/profile.pprof To disable PGO explicitly, use -pgo=off. Handling Multiple Binaries: When building multiple binaries, run separate go build commands if you need to use different profiles for each. Best Practices and Notes Iterative Workflow: Regularly update your profiles and rebuild your application to maintain optimal performance. The typical workflow involves building, collecting profiles, and rebuilding with PGO. Source Stability: Go's PGO is designed to handle changes in your codebase gracefully. Minor changes or refactoring usually do not significantly impact PGO effectiveness. Performance of New Code: Newly added code won't benefit from PGO until new profiles are collected that include its execution. Build Time and Binary Size: Enabling PGO may increase build times due to additional compilation steps. Binary size might slightly increase because of more aggressive inlining and optimizations. Frequently Asked Questions Q1: Can PGO optimize standard library packages and dependencies? Yes, PGO in Go applies to the entire program, including standard library packages and any dependencies your application uses. Q2: Will using an unrepresentative profile make my program slower? Generally, no. While an unrepresentative profile might not provide performance gains, it should not degrade the performance of your application. Q3: Can I use the same profile for different operating systems or architectures? Yes, profiles are compatible across different GOOS/GOARCH settings. However, platform-specific code may not benefit if it differs significantly between platforms. Q4: How should I handle an application used for different workloads? Option 1: Build separate binaries for each workload using profiles specific to each. Option 2: Use profiles from the most critical workload. Option 3: Merge profiles from all workloads to create a general-purpose optimized binary. Q5: How does PGO affect build time and binary size? Enabling PGO can increase build times due to the extra analysis required. Binary sizes may also increase slightly because of additional optimizations like function inlining. Summary Profile-Guided Optimization (PGO) in Go leverages real-world usage data to enhance the performance of Go applications. By collecting CPU profiles from representative runs and feeding them back into the compiler, you enable the compiler to make informed optimization decisions tailored to your application's actual behavior. Steps to Implement PGO in Go: Collect CPU Profiles: Use Go's profiling tools during typical application runs. Prepare the Profile: Save the profile file in the main package directory or specify it with the -pgo flag. Build with PGO: Run go build, and the compiler will use the profile to optimize your application. Key Takeaways: PGO can significantly improve application performance. Regularly updating profiles ensures ongoing optimization benefits. PGO is designed to be robust against code changes and iterative builds. Properly handling profiles when dealing with multiple workloads or platforms is essential for maximizing performance gains. By incorporating PGO into your Go development workflow, you can achieve more efficient and faster applications that better utilize system resources based on actual usage patterns. You can also learn more about it here
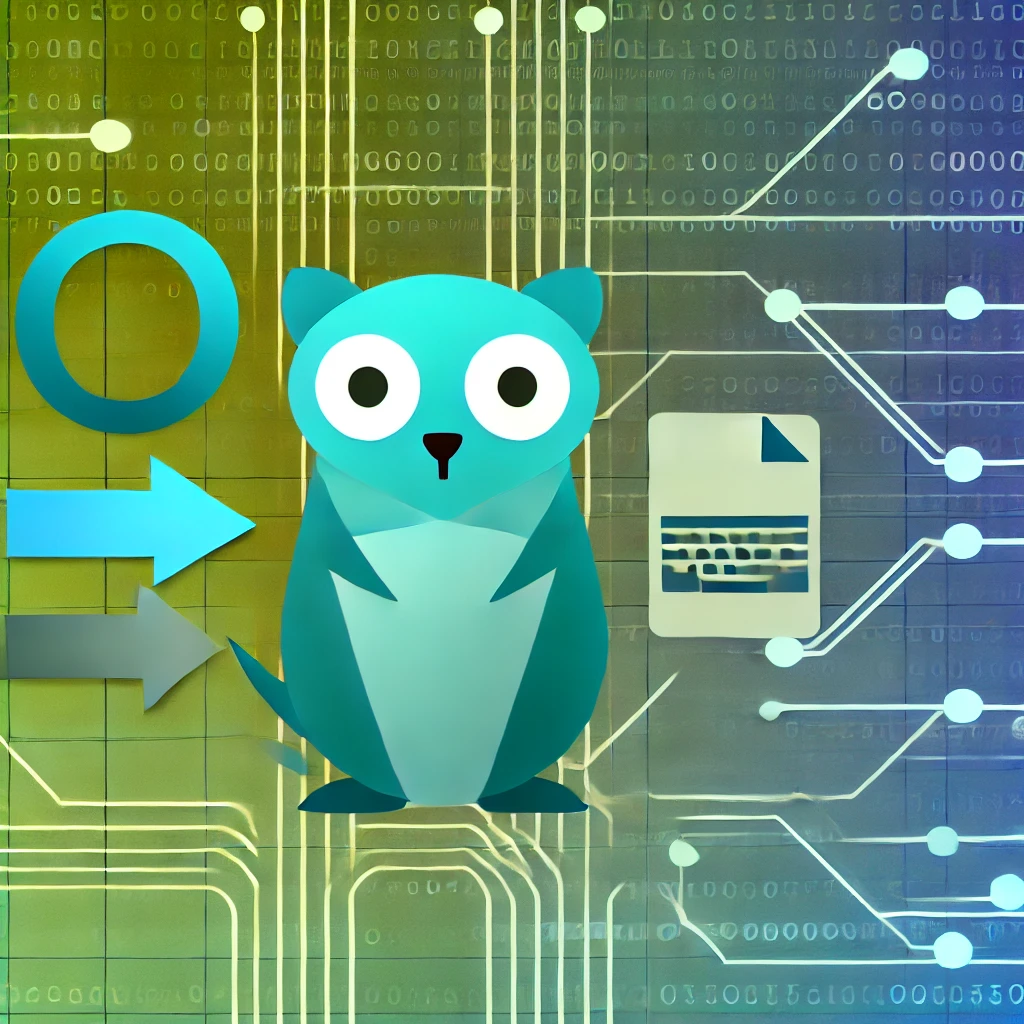
Mastering the io Package in Go: A Detailed Look

Welcome! Building on your understanding of Go's basics and the os package, let's delve into the io package—a cornerstone of Go's standard library. This guide will provide an in-depth exploration of the io package, covering its fundamental interfaces, utility functions, and best practices. Let's get started! Table of Contents Introduction to the io Package Core Interfaces in io Reader Interface Writer Interface Closer Interface Seeker Interface ReadWriter, ReadCloser, WriteCloser, etc. Utility Functions in io Copy and CopyN ReadFull and ReadAtLeast TeeReader MultiReader and MultiWriter Pipe Working with Buffers Using bytes.Buffer Using bufio Package Error Handling in io EOF and Unexpected EOF Sentinel Errors Examples and Use Cases Implementing Custom Readers and Writers Reading from Files, Network Connections, and Strings Writing to Files, Network Connections, and Buffers Best Practices Conclusion Additional Resources Introduction to the io Package The io package in Go provides basic interfaces for I/O primitives, facilitating the reading and writing of byte streams. It is designed to: Abstract data sources and destinations, allowing for flexible and interchangeable components. Provide a common foundation for input/output operations across the standard library and third-party packages. Enable developers to write generic code that can operate on any data stream implementing the core interfaces. By understanding the io package, you'll be able to harness Go's powerful I/O capabilities, write more modular code, and effectively handle data from various sources such as files, network connections, in-memory buffers, and more. Core Interfaces in io Reader Interface The io.Reader interface represents the ability to read data. type Reader interface { Read(p []byte) (n int, err error) } Method: Read(p []byte) (n int, err error): Reads up to len(p) bytes into p. It returns the number of bytes read (n) and any error encountered. Behavior: Blocks until at least one byte is read, an error occurs, or EOF (end-of-file) is reached. Returns n > 0 if any data was read successfully. Returns err == io.EOF when no more data is available. Example Usage: func ReadFromReader(r io.Reader) error { buf := make([]byte, 1024) for { n, err := r.Read(buf) if n > 0 { // Process data in buf[:n] fmt.Printf("Read %d bytes: %s\n", n, string(buf[:n])) } if err != nil { if err == io.EOF { break } return err } } return nil } Writer Interface The io.Writer interface represents the ability to write data. type Writer interface { Write(p []byte) (n int, err error) } Method: Write(p []byte) (n int, err error): Writes len(p) bytes from p to the underlying data stream. Returns the number of bytes written and any error encountered. Behavior: Writes may not accept all bytes in a single call; n may be less than len(p). Should return a non-nil error when n < len(p). Example Usage: func WriteToWriter(w io.Writer, data []byte) error { total := 0 for total < len(data) { n, err := w.Write(data[total:]) if err != nil { return err } total += n } return nil } Closer Interface The io.Closer interface represents the ability to close a data stream. type Closer interface { Close() error } Method: Close() error: Closes the underlying resource, releasing any associated resources. Usage: Commonly used with files, network connections, and other resources that need explicit closure. Seeker Interface The io.Seeker interface allows moving the read/write cursor within a data stream. type Seeker interface { Seek(offset int64, whence int) (int64, error) } Method: Seek(offset int64, whence int) (int64, error): Sets the offset for the next Read or Write to offset, interpreted according to whence. Whence Values: 0 (io.SeekStart): Relative to the origin of the file. 1 (io.SeekCurrent): Relative to the current offset. 2 (io.SeekEnd): Relative to the end. Example Usage: func SeekAndRead(r io.ReadSeeker) error { // Seek to the beginning _, err := r.Seek(0, io.SeekStart) if err != nil { return err } buf := make([]byte, 100) n, err := r.Read(buf) if err != nil { return err } fmt.Printf("Read %d bytes: %s\n", n, string(buf[:n])) return nil } Composed Interfaces ReadWriter Interface type ReadWriter interface { Reader Writer } Represents something that can both read and write. ReadCloser, WriteCloser, ReadWriteCloser io.ReadCloser: Combines io.Reader and io.Closer. io.WriteCloser: Combines io.Writer and io.Closer. io.ReadWriteCloser: Combines io.Reader, io.Writer, and io.Closer. These interfaces are used to represent resources that need to be closed after use, such as files and network connections. Example Usage: func ProcessData(rwc io.ReadWriteCloser) error { defer rwc.Close() // Read from rwc // Write to rwc return nil } Utility Functions in io The io package provides several utility functions that operate on the core interfaces. Copy and CopyN io.Copy() Copies from a Reader to a Writer until EOF or an error occurs. func Copy(dst Writer, src Reader) (written int64, err error) Example Usage: func CopyData(dst io.Writer, src io.Reader) error { _, err := io.Copy(dst, src) return err } io.CopyN() Copies n bytes from a Reader to a Writer. func CopyN(dst Writer, src Reader, n int64) (written int64, err error) ReadFull and ReadAtLeast io.ReadFull() Reads exactly len(buf) bytes from Reader into buf. func ReadFull(r Reader, buf []byte) (n int, err error) Returns io.ErrUnexpectedEOF if the Reader reaches EOF before filling the buffer. io.ReadAtLeast() Reads at least min bytes into buf. func ReadAtLeast(r Reader, buf []byte, min int) (n int, err error) Returns io.ErrUnexpectedEOF if fewer than min bytes are read. TeeReader io.TeeReader returns a Reader that writes to a Writer what it reads from another Reader. func TeeReader(r Reader, w Writer) Reader Use Case: Reading data while simultaneously writing it elsewhere (e.g., logging or hashing input data). Example Usage: func ReadAndLog(r io.Reader) error { var buf bytes.Buffer tee := io.TeeReader(r, &buf) data, err := io.ReadAll(tee) if err != nil { return err } fmt.Println("Original Data:", string(data)) fmt.Println("Logged Data:", buf.String()) return nil } MultiReader and MultiWriter io.MultiReader() Combines multiple Readers into a single Reader. func MultiReader(readers ...Reader) Reader Example Usage: func ConcatenateReaders(r1, r2 io.Reader) io.Reader { return io.MultiReader(r1, r2) } io.MultiWriter() Writes to multiple Writers simultaneously. func MultiWriter(writers ...Writer) Writer Example Usage: func WriteToMultiple(w io.Writer, data []byte) error { mw := io.MultiWriter(os.Stdout, w) _, err := mw.Write(data) return err } Pipe io.Pipe creates a synchronous in-memory pipe. func Pipe() (*PipeReader, *PipeWriter) PipeReader and PipeWriter implement io.Reader and io.Writer respectively. Useful for connecting code expecting a Reader with code expecting a Writer. Example Usage: func UsePipe() error { pr, pw := io.Pipe() go func() { defer pw.Close() pw.Write([]byte("Data sent through pipe")) }() buf := make([]byte, 1024) n, err := pr.Read(buf) if err != nil { return err } fmt.Println("Received:", string(buf[:n])) return nil } Working with Buffers While not part of the io package, bytes.Buffer and bufio are closely related and frequently used with io interfaces. Using bytes.Buffer bytes.Buffer is an in-memory buffer that implements io.Reader, io.Writer, and io.ByteScanner. Example Usage: func UseBuffer() { var buf bytes.Buffer buf.WriteString("Hello, ") buf.Write([]byte("World!")) fmt.Println(buf.String()) // Outputs: Hello, World! // Read from buffer data := make([]byte, buf.Len()) buf.Read(data) fmt.Println(string(data)) // Outputs: Hello, World! } Using bufio Package The bufio package provides buffered Reader and Writer implementations, improving efficiency by reducing the number of system calls. bufio.Reader func UseBufioReader(r io.Reader) { reader := bufio.NewReader(r) line, err := reader.ReadString('\n') if err != nil { // Handle error } fmt.Println("Read line:", line) } bufio.Writer func UseBufioWriter(w io.Writer) { writer := bufio.NewWriter(w) writer.WriteString("Buffered Write\n") writer.Flush() // Ensure data is written to the underlying writer } Error Handling in io EOF and Unexpected EOF io.EOF: Indicates that no more data is available; not considered an error in some contexts. io.ErrUnexpectedEOF: Occurs when EOF is encountered before the expected amount of data is read. Example Handling: n, err := r.Read(buf) if err != nil { if err == io.EOF { // Handle end-of-file condition } else { // Handle other errors } } Sentinel Errors The io package defines several sentinel errors: io.EOF io.ErrClosedPipe io.ErrNoProgress io.ErrShortBuffer io.ErrShortWrite io.ErrUnexpectedEOF These can be compared directly using == to handle specific conditions. Examples and Use Cases Implementing Custom Readers and Writers Custom Reader type MyReader struct { data []byte pos int } func (r *MyReader) Read(p []byte) (n int, err error) { if r.pos >= len(r.data) { return 0, io.EOF } n = copy(p, r.data[r.pos:]) r.pos += n return n, nil } Usage: func main() { r := &MyReader{data: []byte("Hello, Custom Reader!")} buf := make([]byte, 8) for { n, err := r.Read(buf) if n > 0 { fmt.Print(string(buf[:n])) } if err == io.EOF { break } else if err != nil { fmt.Println("Error:", err) break } } } Custom Writer type MyWriter struct { data []byte } func (w *MyWriter) Write(p []byte) (n int, err error) { w.data = append(w.data, p...) return len(p), nil } Usage: func main() { w := &MyWriter{} w.Write([]byte("Hello, ")) w.Write([]byte("Custom Writer!")) fmt.Println(string(w.data)) // Outputs: Hello, Custom Writer! } Reading from Various Sources Reading from a File func ReadFromFile(filename string) error { file, err := os.Open(filename) if err != nil { return err } defer file.Close() return ReadFromReader(file) } Reading from a Network Connection func ReadFromConnection(conn net.Conn) error { defer conn.Close() return ReadFromReader(conn) } Reading from a String Use strings.NewReader to create a Reader from a string. func ReadFromString(s string) error { r := strings.NewReader(s) return ReadFromReader(r) } Writing to Various Destinations Writing to a File func WriteToFile(filename string, data []byte) error { file, err := os.Create(filename) if err != nil { return err } defer file.Close() return WriteToWriter(file, data) } Writing to a Network Connection func WriteToConnection(conn net.Conn, data []byte) error { defer conn.Close() return WriteToWriter(conn, data) } Writing to a Buffer func WriteToBuffer(data []byte) (*bytes.Buffer, error) { var buf bytes.Buffer err := WriteToWriter(&buf, data) if err != nil { return nil, err } return &buf, nil } Best Practices Use Interfaces for Abstraction: Accept io.Reader and io.Writer in function parameters to allow for flexibility and testability. Return interfaces when appropriate to abstract implementation details. Handle Errors Properly: Always check and handle errors returned by Read, Write, and other methods. Be mindful of partial reads and writes. Buffering for Efficiency: Use bufio.Reader and bufio.Writer when dealing with small reads and writes to improve performance. Close Resources: Close any resources that implement io.Closer using defer to ensure they are released properly. Understand Blocking Behavior: Be aware that Read and Write operations may block, especially when dealing with network connections. Use Utility Functions: Leverage functions like io.Copy, io.CopyN, io.ReadFull, and others to simplify code and handle common patterns. Implement Interfaces Thoughtfully: When creating custom types that implement io interfaces, ensure they conform to the expected behaviors and handle edge cases. Avoid Unnecessary Data Copies: When possible, operate directly on streams to minimize memory usage and improve performance. Document Behavior: Clearly document how your functions and types interact with the io interfaces, especially regarding error handling and partial reads/writes. Conclusion The io package is fundamental to Go's approach to input and output operations. By mastering its interfaces and utilities, you can write flexible, efficient, and modular code that interacts seamlessly with various data sources and destinations. Understanding the io package enables you to: Build applications that can read from and write to files, network connections, buffers, and more. Implement custom readers and writers tailored to your application's needs. Leverage powerful utilities to simplify data transfer and processing. Write code that is easy to test and maintain due to its adherence to standard interfaces. Additional Resources Official Documentation: io package documentation bufio package documentation bytes package documentation Go by Example: Reading Files Writing Files Line Filters Pipes Blogs and Tutorials: An Introduction to the io.Reader Interface in Go Understanding Golang io.Reader and io.Writer Working Effectively with Go's io.Reader and io.Writer Interfaces Books: The Go Programming Language by Alan A. A. Donovan and Brian W. Kernighan (Chapter on Interfaces) Keep experimenting with the io package and its interfaces to deepen your understanding and enhance your Go programming expertise! By doing so, you'll be well-equipped to build robust, efficient, and scalable applications.
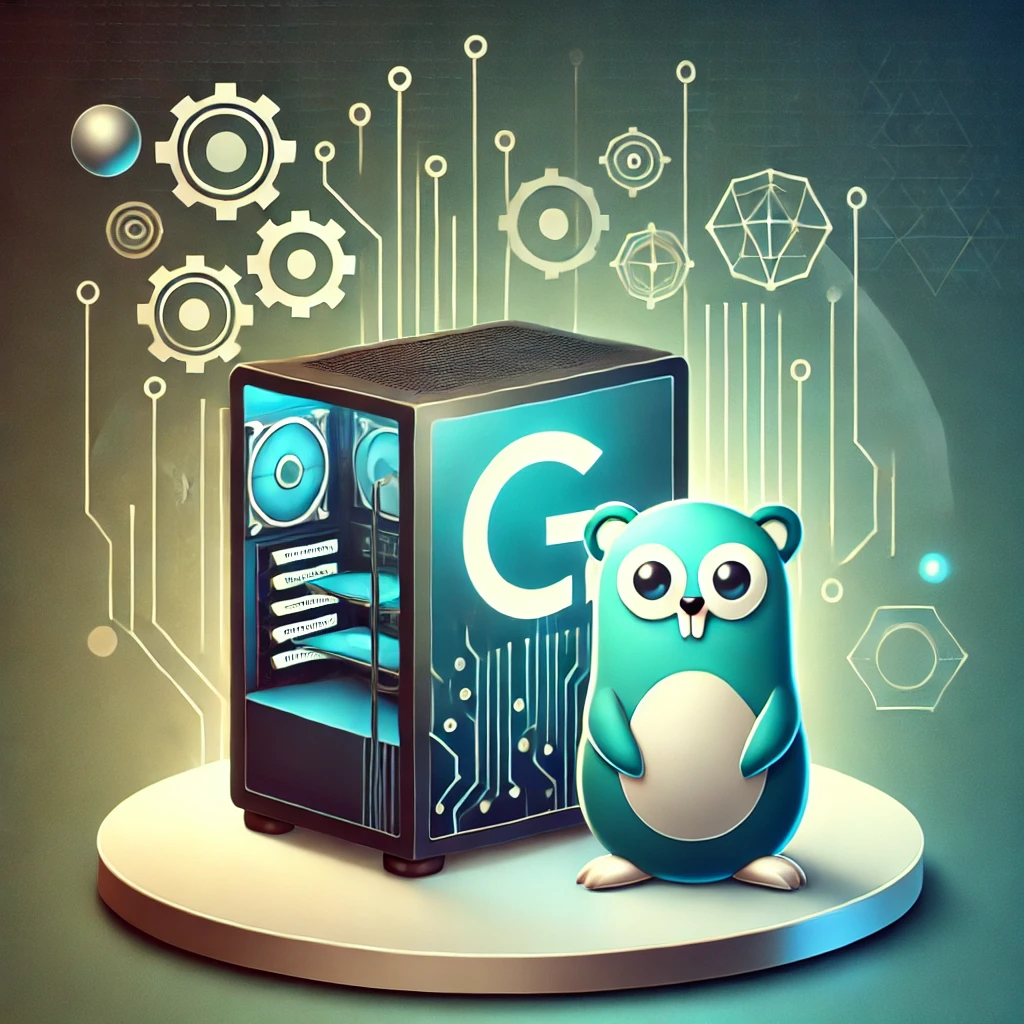
Understanding the os Package in Go Programming Language

Welcome! Now that you've grasped the basics of Go, let's delve deeper into one of its most essential packages: the os package. This guide will provide an in-depth exploration of the os package, covering everything from file operations to process management. Let's get started! Table of Contents Introduction to the os Package Importing the os Package Working with Files Opening and Creating Files Reading and Writing Files Closing Files File Permissions and Modes Working with Directories Creating Directories Listing Directory Contents Removing Files and Directories File Information Getting File Info FileInfo Interface Environment Variables Getting Environment Variables Setting Environment Variables Listing All Environment Variables Process Management Process IDs and Exit Codes Executing External Commands Signals Handling Signals Temporary Files and Directories Symlinks and File System Operations Constants and Variables in os Best Practices Conclusion Additional Resources Introduction to the os Package The os package in Go provides a platform-independent interface to operating system functionality. It allows you to perform: File and directory operations Process and signal handling Environment variable manipulation File permission and ownership management Interactions with the underlying file system The os package abstracts differences between operating systems, enabling you to write code that works across Windows, macOS, Linux, and other supported platforms. Importing the os Package To use the os package, import it in your Go program: import "os" Optionally, you may import other related packages like fmt, io, or path/filepath as needed. Working with Files Opening and Creating Files Opening Existing Files Use os.Open() to open an existing file for reading. file, err := os.Open("example.txt") if err != nil { // Handle error fmt.Println("Error opening file:", err) return } defer file.Close() Creating or Truncating Files Use os.Create() to create a new file or truncate an existing one. file, err := os.Create("newfile.txt") if err != nil { // Handle error fmt.Println("Error creating file:", err) return } defer file.Close() Opening Files with Specific Flags and Permissions Use os.OpenFile() to open a file with specific flags and permissions. file, err := os.OpenFile("example.txt", os.O_RDWR|os.O_CREATE, 0755) if err != nil { // Handle error fmt.Println("Error opening file:", err) return } defer file.Close() Flags: os.O_RDONLY: Open the file read-only. os.O_WRONLY: Open the file write-only. os.O_RDWR: Open the file read-write. os.O_APPEND: Append data to the file when writing. os.O_CREATE: Create a new file if none exists. os.O_TRUNC: Truncate file when opened. Permissions: File mode bits (e.g., 0644, 0755) specify the file's permission and mode bits. Reading and Writing Files Reading from Files Use methods from the io package or os.File methods to read data. Using Read() method: buffer := make([]byte, 100) bytesRead, err := file.Read(buffer) if err != nil { // Handle error fmt.Println("Error reading file:", err) return } fmt.Printf("Read %d bytes: %s\n", bytesRead, string(buffer[:bytesRead])) Using bufio package: import ( "bufio" // ... ) scanner := bufio.NewScanner(file) for scanner.Scan() { fmt.Println(scanner.Text()) } if err := scanner.Err(); err != nil { fmt.Println("Error scanning file:", err) } Writing to Files Use Write() or WriteString() methods. data := []byte("Hello, World!\n") bytesWritten, err := file.Write(data) if err != nil { // Handle error fmt.Println("Error writing to file:", err) return } fmt.Printf("Wrote %d bytes to file.\n", bytesWritten) Using fmt.Fprintf(): import "fmt" fmt.Fprintf(file, "Formatted number: %d\n", 42) Closing Files Always close files when done to release resources. defer file.Close() Using defer ensures that the file is closed when the function exits, even if an error occurs. File Permissions and Modes File permissions are specified using Unix-style permission bits. Common Permission Bits: 0644: Owner can read and write; others can read. 0755: Owner can read, write, and execute; others can read and execute. Example: file, err := os.OpenFile("script.sh", os.O_CREATE|os.O_WRONLY, 0755) Working with Directories Creating Directories Create a Single Directory Use os.Mkdir() to create a single directory. err := os.Mkdir("testdir", 0755) if err != nil { fmt.Println("Error creating directory:", err) return } Create Nested Directories Use os.MkdirAll() to create nested directories. err := os.MkdirAll("parent/child/grandchild", 0755) if err != nil { fmt.Println("Error creating directories:", err) return } Listing Directory Contents Use os.ReadDir() (Go 1.16 and later) or ioutil.ReadDir() (deprecated in Go 1.16) to list directory contents. Using os.ReadDir(): entries, err := os.ReadDir(".") if err != nil { fmt.Println("Error reading directory:", err) return } for _, entry := range entries { fmt.Println(entry.Name()) } Entry Attributes: entry.Name(): Name of the file or directory. entry.IsDir(): Returns true if the entry is a directory. Removing Files and Directories Remove a File Use os.Remove(). err := os.Remove("oldfile.txt") if err != nil { fmt.Println("Error removing file:", err) return } Remove a Directory Use os.Remove() if the directory is empty. err := os.Remove("emptydir") Remove a Directory and Its Contents Use os.RemoveAll(). err := os.RemoveAll("parent") if err != nil { fmt.Println("Error removing directory and its contents:", err) return } File Information Getting File Info Use os.Stat() or os.Lstat() to get file information. info, err := os.Stat("example.txt") if err != nil { if os.IsNotExist(err) { fmt.Println("File does not exist.") } else { fmt.Println("Error stating file:", err) } return } fmt.Printf("File Name: %s\n", info.Name()) fmt.Printf("Size: %d bytes\n", info.Size()) fmt.Printf("Permissions: %s\n", info.Mode()) fmt.Printf("Modification Time: %s\n", info.ModTime()) fmt.Printf("Is Directory: %t\n", info.IsDir()) FileInfo Interface os.FileInfo is an interface providing methods to access file metadata. Methods: Name() string: Base name of the file. Size() int64: Length in bytes. Mode() FileMode: File mode bits. ModTime() time.Time: Modification time. IsDir() bool: Returns true if the file is a directory. Sys() interface{}: Underlying data source (can be used for platform-specific information). Environment Variables Getting Environment Variables Use os.Getenv() to get the value of an environment variable. path := os.Getenv("PATH") fmt.Println("PATH:", path) Setting Environment Variables Use os.Setenv() to set an environment variable. err := os.Setenv("MY_VAR", "my_value") if err != nil { fmt.Println("Error setting environment variable:", err) return } Unsetting Environment Variables Use os.Unsetenv() to remove an environment variable. err := os.Unsetenv("MY_VAR") if err != nil { fmt.Println("Error unsetting environment variable:", err) return } Listing All Environment Variables Use os.Environ() to get a slice of all environment variables in the format "KEY=value". envVars := os.Environ() for _, envVar := range envVars { fmt.Println(envVar) } To split the key and value: for _, envVar := range envVars { pair := strings.SplitN(envVar, "=", 2) key := pair[0] value := pair[1] fmt.Printf("%s: %s\n", key, value) } Process Management Process IDs and Exit Codes Getting the Current Process ID pid := os.Getpid() fmt.Println("Process ID:", pid) Getting the Parent Process ID ppid := os.Getppid() fmt.Println("Parent Process ID:", ppid) Exiting the Program with a Status Code Use os.Exit(). func main() { // ... if errorOccurred { fmt.Println("An error occurred.") os.Exit(1) // Non-zero exit code indicates an error } os.Exit(0) // Zero exit code indicates success } Note: Deferred functions are not run when os.Exit() is called. Executing External Commands For executing external commands, use the os/exec package. import ( "os/exec" // ... ) cmd := exec.Command("ls", "-l") output, err := cmd.Output() if err != nil { fmt.Println("Error executing command:", err) return } fmt.Println(string(output)) Signals Handling Signals Use the os/signal package to handle operating system signals. import ( "os" "os/signal" "syscall" // ... ) func main() { sigs := make(chan os.Signal, 1) done := make(chan bool, 1) signal.Notify(sigs, syscall.SIGINT, syscall.SIGTERM) go func() { sig := <-sigs fmt.Println() fmt.Println("Received signal:", sig) done <- true }() fmt.Println("Awaiting signal") <-done fmt.Println("Exiting") } This program waits for SIGINT (Ctrl+C) or SIGTERM signals and exits gracefully when received. Temporary Files and Directories Creating Temporary Files Use os.CreateTemp() (Go 1.17 and later) or ioutil.TempFile() (deprecated) to create a temporary file. file, err := os.CreateTemp("", "tempfile_*.txt") if err != nil { fmt.Println("Error creating temporary file:", err) return } defer os.Remove(file.Name()) // Clean up fmt.Println("Temporary file created:", file.Name()) Creating Temporary Directories Use os.MkdirTemp() (Go 1.17 and later) or ioutil.TempDir() (deprecated). dir, err := os.MkdirTemp("", "tempdir_") if err != nil { fmt.Println("Error creating temporary directory:", err) return } defer os.RemoveAll(dir) // Clean up fmt.Println("Temporary directory created:", dir) Symlinks and File System Operations Creating Symlinks Use os.Symlink(). err := os.Symlink("target.txt", "link.txt") if err != nil { fmt.Println("Error creating symlink:", err) return } Reading Symlinks Use os.Readlink(). target, err := os.Readlink("link.txt") if err != nil { fmt.Println("Error reading symlink:", err) return } fmt.Println("Symlink points to:", target) Changing File Permissions Use os.Chmod(). err := os.Chmod("example.txt", 0644) if err != nil { fmt.Println("Error changing file permissions:", err) return } Changing File Ownership Use os.Chown(). err := os.Chown("example.txt", uid, gid) if err != nil { fmt.Println("Error changing file ownership:", err) return } Note: Changing ownership may require elevated privileges. Renaming and Moving Files Use os.Rename(). err := os.Rename("oldname.txt", "newname.txt") if err != nil { fmt.Println("Error renaming file:", err) return } Copying Files The os package does not provide a direct method to copy files. You can read from the source and write to the destination. func copyFile(src, dst string) error { sourceFile, err := os.Open(src) if err != nil { return err } defer sourceFile.Close() destFile, err := os.Create(dst) if err != nil { return err } defer destFile.Close() _, err = io.Copy(destFile, sourceFile) if err != nil { return err } // Flush file to storage err = destFile.Sync() return err } Constants and Variables in os File Mode Constants The os package provides constants to represent file modes and permissions. File Types: os.ModeDir: Is a directory. os.ModeSymlink: Is a symbolic link. os.ModeNamedPipe: Is a named pipe (FIFO). os.ModeSocket: Is a Unix domain socket. os.ModeDevice: Is a device file. Permission Bits: os.ModePerm: 0777, Unix permission bits. Common Variables os.PathSeparator: Platform-specific path separator ('/' on Unix, '\' on Windows). os.PathListSeparator: Separator for list of paths (':' on Unix, ';' on Windows). os.DevNull: Name of the null device ("/dev/null" on Unix, "NUL" on Windows). Best Practices Error Handling: Always check for errors when performing file and directory operations. Resource Management: Use defer to ensure files are closed and resources are released. Platform Independence: Be cautious of platform-specific behavior. Use filepath package for manipulating file paths in a platform-independent way. Permissions: Be mindful of file permissions, especially when creating files and directories. Security Considerations: Validate inputs when dealing with file paths to prevent path traversal vulnerabilities. Concurrency: Be cautious when accessing files from multiple goroutines. Use synchronization mechanisms if necessary. Conclusion The os package is a powerful tool for interacting with the operating system in Go. It provides a rich set of functions for file and directory manipulation, environment variable management, process control, and more. Understanding how to use the os package effectively is essential for building robust and efficient applications. By mastering the os package, you'll be able to: Read and write files efficiently. Manage file permissions and ownership. Navigate and manipulate the file system. Handle environment variables and configurations. Interact with processes and handle signals. Create utilities and tools that require low-level OS interactions. Additional Resources Official Documentation: os package documentation io package documentation path/filepath package documentation os/exec package documentation os/signal package documentation Go by Example: File Paths Reading Files Writing Files Environment Variables Signals Blogs and Tutorials: File Handling in Go Working with Directories in Go Keep exploring and experimenting with the os package to deepen your understanding and enhance your Go programming skills!

How to Build a CI/CD Pipeline with ArgoCD on Civo Kubernetes

In this blog post, we will build a complete production-ready CI/CD setup for deploying microservices using ArgoCD on a Civo Kubernetes cluster. We will also integrate PostgreSQL using Persistent Volumes (PV) and Persistent Volume Claims (PVC) to ensure our database remains persistent. Prerequisites A running Civo Kubernetes cluster (if you don’t have one, check out the Civo CLI to create one). Basic knowledge of Kubernetes, Docker, and GitOps. Docker Hub account (for pushing your microservice images). PostgreSQL and Kubernetes basics. ArgoCD installed on your Civo Kubernetes cluster (we will cover the installation below). Step 1: Installing ArgoCD on Civo Kubernetes Cluster First, we need to install ArgoCD to manage our application deployments using GitOps principles. 1.1 Install ArgoCD Execute the following commands to install ArgoCD: kubectl create namespace argocd kubectl apply -n argocd -f https://raw.githubusercontent.com/argoproj/argo-cd/stable/manifests/install.yaml 1.2 Access ArgoCD UI ArgoCD comes with a web-based UI to manage deployments. To access it: Set up port forwarding: kubectl port-forward svc/argocd-server -n argocd 8080:443 Open a browser and navigate to https://localhost:8080 (if did not work,move to incognito mode and then go to localhost:8080). You will need to log in with the default admin user: Get the initial admin password: kubectl get secret argocd-initial-admin-secret -n argocd -o jsonpath="{.data.password}" | base64 -d Username: admin You now have access to the ArgoCD UI where you can manage your applications. Step 2: Setting Up GitOps Workflow for Microservices In this step, we’ll configure a user-service as a microservice. The microservice uses PostgreSQL for its database and connects to it via environment variables. 2.1 Preparing Kubernetes Manifests for user-service We will define a user-service deployment and service. Additionally, we will set up PostgreSQL with a persistent volume claim (PVC) for persistent storage. Create the following YAML files and commit them to your Git repository. 2.1.1 user-service-deployment.yaml apiVersion: apps/v1 kind: Deployment metadata: name: user-service labels: app: user-service spec: replicas: 3 selector: matchLabels: app: user-service template: metadata: labels: app: user-service spec: containers: - name: user-service image: <your-docker-image> ports: - containerPort: 8081 env: - name: POSTGRES_USER valueFrom: secretKeyRef: name: postgres-secret key: POSTGRES_USER - name: POSTGRES_PASSWORD valueFrom: secretKeyRef: name: postgres-secret key: POSTGRES_PASSWORD - name: POSTGRES_DB value: "user_db" - name: POSTGRES_HOST value: "postgres-service" 2.1.2 user-service-service.yaml apiVersion: v1 kind: Service metadata: name: user-service spec: selector: app: user-service ports: - protocol: TCP port: 80 targetPort: 8081 type: ClusterIP Step 3: PostgreSQL with Persistent Storage 3.1 PostgreSQL Deployment and PVC Next, we'll define PostgreSQL and link it with a Persistent Volume Claim (PVC) to ensure data persists even if the PostgreSQL pod restarts. 3.1.1 postgres-deployment.yaml apiVersion: v1 kind: Secret metadata: name: postgres-secret type: Opaque data: POSTGRES_USER: cG9zdGdyZXM= # base64 encoded "postgres" POSTGRES_PASSWORD: cGFzc3dvcmQ= # base64 encoded "password" --- apiVersion: apps/v1 kind: Deployment metadata: name: postgres labels: app: postgres spec: replicas: 1 selector: matchLabels: app: postgres template: metadata: labels: app: postgres spec: containers: - name: postgres image: postgres:14 env: - name: POSTGRES_USER valueFrom: secretKeyRef: name: postgres-secret key: POSTGRES_USER - name: POSTGRES_PASSWORD valueFrom: secretKeyRef: name: postgres-secret key: POSTGRES_PASSWORD - name: POSTGRES_DB value: "user_db" ports: - containerPort: 5432 volumeMounts: - name: postgres-data mountPath: /var/lib/postgresql/data volumes: - name: postgres-data persistentVolumeClaim: claimName: postgres-pv-claim 3.1.2 postgres-pv.yaml apiVersion: v1 kind: PersistentVolumeClaim metadata: name: postgres-pv-claim spec: accessModes: - ReadWriteOnce resources: requests: storage: 10Gi --- apiVersion: v1 kind: Service metadata: name: postgres-service spec: ports: - port: 5432 targetPort: 5432 selector: app: postgres type: ClusterIP This configuration ensures that the PostgreSQL pod has persistent storage for the database. Step 4: Setting Up ArgoCD Application Now, we need to tell ArgoCD to monitor our Git repository and automatically sync our application to Kubernetes whenever changes are made. 4.1 Create ArgoCD Application We’ll create an ArgoCD application for the user-service using the following YAML file. 4.1.1 user-service-argocd-application.yaml apiVersion: argoproj.io/v1alpha1 kind: Application metadata: name: user-service namespace: argocd spec: destination: namespace: default server: https://kubernetes.default.svc source: repoURL: 'https://github.com/yourusername/user-service-repo.git' targetRevision: HEAD path: manifests project: default syncPolicy: automated: prune: true selfHeal: true 4.2 Apply the ArgoCD Application Once the YAML file is ready, apply it to your cluster: kubectl apply -f user-service-argocd-application.yaml This will instruct ArgoCD to automatically sync your Kubernetes manifests from your Git repository. Step 5: Setting Up Ingress (Optional) To expose your microservice, create an ingress resource and secure it with TLS (Let’s Encrypt). Here’s a sample ingress configuration: apiVersion: networking.k8s.io/v1 kind: Ingress metadata: name: user-ingress annotations: cert-manager.io/cluster-issuer: "letsencrypt-prod" spec: tls: - hosts: - your-domain.com secretName: tls-secret rules: - host: your-domain.com http: paths: - path: / pathType: Prefix backend: service: name: user-service port: number: 80 Step 6: Testing and Monitoring with ArgoCD Push Changes to GitHub: Modify your Kubernetes manifests and push them to your Git repository. Observe Sync in ArgoCD UI: Head over to the ArgoCD UI to see the synchronization of your application in action. Deployment: Your Kubernetes cluster will automatically deploy or update the application based on changes pushed to the Git repository. Conclusion With this setup, you now have a fully production-ready CI/CD pipeline using ArgoCD on Civo Kubernetes. Every time you push changes to your Kubernetes manifests in your Git repository, ArgoCD will automatically sync those changes and ensure your application state in the cluster matches the desired state in Git. Additionally, PostgreSQL is configured with a Persistent Volume for persistent data, ensuring the database survives pod restarts. Feel free to extend this setup with more microservices, monitoring tools like Prometheus, and alerting systems as needed for your production environment. Happy deploying with ArgoCD!
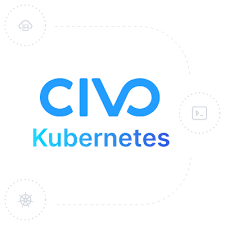
Setup a Kubernetes cluster on Civo

Setting Up a Civo Kubernetes Cluster Using the CLI: A Step-by-Step Guide If you're looking to create a Kubernetes cluster quickly and easily using Civo, you're in the right place. This guide will walk you through the steps to set up a Kubernetes cluster using the Civo CLI and get your environment up and running. Step 1: Install Civo CLI Before you start, make sure you have the Civo CLI installed on your local machine. You can do this by following the installation instructions in the official Civo CLI documentation. You can verify the installation by running: civo --version Step 2: Install kubectl You also need to have kubectl installed for managing your Kubernetes clusters. You can install it by following the instructions on the official kubectl documentation. Once installed, verify it by running: kubectl version --client Step 3: Create a Civo Account and Start a Kubernetes Cluster Head over to Civo's dashboard and create an account if you don’t already have one. Navigate to the Kubernetes section and create a new Kubernetes cluster. Once the cluster is up and running, generate an API key to interact with the Civo CLI. You can find this in your Account settings. Step 4: Save Your API Key With your API key created, it’s time to configure the Civo CLI to recognize it. Run the following command: civo apikey save <your-api-key-name> The CLI will prompt you to paste your API key. Once done, your CLI will be authorized to manage your clusters. Note: You can also create a Kubernetes Cluster using civo cli Step 5: Get the Kubeconfig File After the cluster is created, download the kubeconfig file from your Civo dashboard. This file allows kubectl to interact with your Kubernetes cluster. Step 6: Set the Kubeconfig Environment Variable You need to tell kubectl where to find your kubeconfig file. Run the following command, replacing <path/to/your/kubeconfig/file> with the actual path to your kubeconfig file: export KUBECONFIG=<path/to/your/kubeconfig/file> Step 7: Verify the Cluster Setup To confirm that the Kubernetes cluster is set up properly, run: kubectl get nodes If this command runs successfully and you see a list of nodes, congratulations! You have successfully configured your Civo Kubernetes cluster. Conclusion Setting up a Kubernetes cluster with Civo CLI is a straightforward process that simplifies getting started with Kubernetes. With just a few steps, you can spin up a cluster and start deploying your applications. Happy coding!
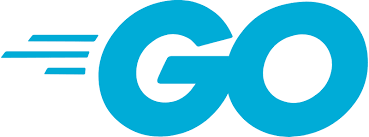
Understanding the Fundamentals of Go Programming

Welcome to the world of Go! This guide is designed to prepare you thoroughly for the basics of Go programming, covering all fundamental concepts. Let's dive in! Table of Contents Introduction to Go Setting Up the Go Environment Basic Syntax and Structure Variables and Data Types Constants Operators Control Structures If-Else Statements Switch Statements For Loops Functions Variadic Functions Anonymous Functions Closures Deferred Function Calls Pointers Arrays and Slices Maps Structs Methods Embedding (Composition) Interfaces Error Handling Concurrency Goroutines Channels Packages and Modules Standard Library Overview Testing Best Practices Conclusion Introduction to Go Go, also known as Golang, is an open-source programming language developed by Google. It is designed for building fast, reliable, and efficient software. Key features include: Static Typing and Efficiency: Go is statically typed and compiles to native machine code. Concurrency Support: Built-in support for concurrent programming with goroutines and channels. Simplicity and Readability: A clean syntax inspired by C, but with modern features. Garbage Collection: Automatic memory management. Setting Up the Go Environment Download and Install Go Download Go: Visit the official Go website and download the installer for your operating system. Install Go: Run the installer and follow the prompts. Verify Installation: Open a terminal or command prompt and run: go version You should see the installed Go version. Setting Up GOPATH and GOROOT GOROOT: The location where Go is installed. It's usually set automatically. GOPATH: The workspace directory where your Go projects and dependencies reside. Set GOPATH environment variable to your workspace directory, e.g., ~/go on Unix systems. Hello World Example Create a file named main.go: package main import "fmt" func main() { fmt.Println("Hello, World!") } Run the program: go run main.go Basic Syntax and Structure Packages: Every Go file belongs to a package. Imports: Use import to include packages. Functions: The main function is the entry point. Example: package main import "fmt" func main() { fmt.Println("Go Basics") } Variables and Data Types Declaring Variables Using var keyword: var a int = 10 var b = 20 // Type inferred as int var c string Short Variable Declaration: d := 30 // Type inferred as int Data Types Boolean: bool Numeric: Integers: int, int8, int16, int32, int64, uint (unsigned) Floating-point: float32, float64 Complex numbers: complex64, complex128 String: string Byte and Rune: byte (alias for uint8) rune (alias for int32, represents Unicode code points) Zero Values Variables declared without an explicit initial value are given their zero value: Numeric types: 0 Boolean: false String: "" (empty string) Pointers, functions, interfaces, slices, channels, maps: nil Constants Defined using the const keyword. const Pi = 3.14159 const Greeting = "Hello, World!" Constants cannot be declared using the := syntax. Operators Arithmetic Operators: +, -, *, /, % Assignment Operators: =, +=, -=, *=, /=, %= Comparison Operators: ==, !=, >, <, >=, <= Logical Operators: &&, ||, ! Bitwise Operators: &, |, ^, &^, <<, >> Control Structures If-Else Statements if x > 10 { fmt.Println("x is greater than 10") } else if x == 10 { fmt.Println("x is equal to 10") } else { fmt.Println("x is less than 10") } Short Statement in If: if err := doSomething(); err != nil { fmt.Println("Error:", err) } Switch Statements switch day { case "Monday": fmt.Println("Start of the work week.") case "Friday": fmt.Println("End of the work week.") default: fmt.Println("Midweek days.") } Switch with No Expression: switch { case x > 0: fmt.Println("x is positive") case x < 0: fmt.Println("x is negative") default: fmt.Println("x is zero") } For Loops Basic For Loop: for i := 0; i < 5; i++ { fmt.Println(i) } While Loop Equivalent: i := 0 for i < 5 { fmt.Println(i) i++ } Infinite Loop: for { // Do something } Range in For Loop: nums := []int{2, 4, 6} for index, value := range nums { fmt.Printf("Index: %d, Value: %d\n", index, value) } Functions Defining Functions func functionName(parameterName parameterType) returnType { // Function body return value } Example: func add(a int, b int) int { return a + b } Multiple Return Values func divide(a, b int) (int, int) { quotient := a / b remainder := a % b return quotient, remainder } Named Return Values func split(sum int) (x, y int) { x = sum * 4 / 9 y = sum - x return // Returns x, y } Variadic Functions Functions that accept a variable number of arguments. func sum(nums ...int) int { total := 0 for _, num := range nums { total += num } return total } Anonymous Functions func() { fmt.Println("Anonymous function") }() Closures Functions that reference variables from outside their body. func adder() func(int) int { sum := 0 return func(x int) int { sum += x return sum } } func main() { pos, neg := adder(), adder() fmt.Println(pos(1)) // 1 fmt.Println(pos(2)) // 3 fmt.Println(neg(-1)) // -1 } Deferred Function Calls Deferred functions are executed after the surrounding function returns. func main() { defer fmt.Println("World") fmt.Println("Hello") } // Output: // Hello // World Pointers Pointers store the memory address of a value. var x int = 10 var p *int = &x // p points to x fmt.Println(*p) // Dereferencing p gives the value of x *p = 20 // Modifies x through the pointer fmt.Println(x) // x is now 20 Nil Pointer: A pointer with zero value nil does not point to any address. Arrays and Slices Arrays Fixed-size sequences of elements of a single type. var arr [5]int // An array of 5 integers arr := [3]string{"a", "b", "c"} Slices Dynamic-size, flexible view into arrays. var s []int // A slice of integers s = arr[1:3] // Slice from array // Creating slices s := []int{1, 2, 3} // Append to slices s = append(s, 4, 5) // Slice capacity and length fmt.Println(len(s), cap(s)) Slice Internals Length: Number of elements in the slice. Capacity: Number of elements in the underlying array starting from the slice's first element. Maps Maps are unordered collections of key-value pairs. m := make(map[string]int) m["one"] = 1 m["two"] = 2 // Accessing values value := m["one"] // Checking for key existence value, exists := m["three"] if !exists { fmt.Println("Key not found") } // Deleting keys delete(m, "two") Structs Custom data types that group together fields. type Person struct { Name string Age int } func main() { p := Person{Name: "Alice", Age: 30} fmt.Println(p.Name) } Methods Functions associated with a type. func (p Person) Greet() { fmt.Printf("Hello, my name is %s.\n", p.Name) } func main() { p := Person{Name: "Bob"} p.Greet() } Pointer Receivers Methods can have pointer receivers to modify the struct. func (p *Person) HaveBirthday() { p.Age++ } Embedding (Composition) One struct can embed another, promoting code reuse. type Employee struct { Person EmployeeID string } func main() { e := Employee{ Person: Person{Name: "Carol", Age: 28}, EmployeeID: "E123", } e.Greet() // Inherits Greet from Person } Interfaces Interfaces define a set of method signatures. type Shape interface { Area() float64 } type Circle struct { Radius float64 } func (c Circle) Area() float64 { return math.Pi * c.Radius * c.Radius } type Rectangle struct { Width, Height float64 } func (r Rectangle) Area() float64 { return r.Width * r.Height } func main() { var s Shape s = Circle{Radius: 5} fmt.Println("Circle Area:", s.Area()) s = Rectangle{Width: 4, Height: 6} fmt.Println("Rectangle Area:", s.Area()) } Empty Interface: interface{} can hold any type. Type Assertions To extract the underlying value from an interface. var i interface{} = "Hello" s := i.(string) fmt.Println(s) Type Switches: switch v := i.(type) { case string: fmt.Println("String:", v) case int: fmt.Println("Integer:", v) default: fmt.Println("Unknown type") } Error Handling In Go, errors are values. func doSomething() error { // ... return errors.New("Something went wrong") } func main() { if err := doSomething(); err != nil { fmt.Println("Error:", err) } } Custom Error Types Implement the error interface. type MyError struct { Code int Message string } func (e *MyError) Error() string { return fmt.Sprintf("Error %d: %s", e.Code, e.Message) } Concurrency Goroutines Lightweight threads managed by Go runtime. func say(s string) { fmt.Println(s) } func main() { go say("Hello") go say("World") // Wait for goroutines to finish time.Sleep(time.Second) } Channels Channels are used for communication between goroutines. c := make(chan int) // Sending and receiving go func() { c <- 42 // Send 42 to channel c }() value := <-c // Receive from channel c fmt.Println(value) // Outputs: 42 Buffered Channels: c := make(chan int, 2) c <- 1 c <- 2 Channel Direction: func send(c chan<- int, value int) { c <- value } func receive(c <-chan int) { value := <-c fmt.Println(value) } Select Statement Waits on multiple channel operations. func fibonacci(c, quit chan int) { x, y := 0, 1 for { select { case c <- x: x, y = y, x+y case <-quit: fmt.Println("Quit") return } } } func main() { c := make(chan int) quit := make(chan int) go func() { for i := 0; i < 10; i++ { fmt.Println(<-c) } quit <- 0 }() fibonacci(c, quit) } Packages and Modules Packages Code organization in Go is done using packages. Every Go file starts with package declaration. Importing Packages import ( "fmt" "math" ) Modules Modules are collections of packages. Initialize a module: go mod init module-name Add dependencies: go get package-path Standard Library Overview Go's standard library provides a rich set of packages: fmt: Formatting I/O os: Operating system functionality io: Basic interfaces to I/O primitives bufio: Buffered I/O net/http: HTTP client and server implementations strconv: String conversions encoding/json: JSON encoding and decoding time: Time functionality math: Basic math functions sync: Synchronization primitives like mutexes context: Context propagation for cancellation, timeouts Testing Go has built-in support for testing. Writing Tests Create a file ending with _test.go. package math import "testing" func TestAdd(t *testing.T) { total := Add(2, 3) if total != 5 { t.Errorf("Add(2, 3) = %d; want 5", total) } } Run tests: go test Benchmarking func BenchmarkAdd(b *testing.B) { for i := 0; i < b.N; i++ { Add(2, 3) } } Best Practices Code Formatting: Use gofmt or go fmt to format code. Naming Conventions: Use CamelCase for functions and variable names. Error Handling: Check errors explicitly. Avoid Global Variables: Use local variables or pass variables as parameters. Write Tests: Ensure code reliability. Documentation: Comment your code and use godoc for documentation. Conclusion You've now covered the fundamental concepts of Go programming, from basic syntax to advanced topics like concurrency and interfaces. Go's simplicity and powerful features make it an excellent choice for building efficient and scalable software. Next Steps: Practice: Build small projects to apply what you've learned. Explore Packages: Delve deeper into the standard library. Concurrency Patterns: Study concurrency patterns for efficient goroutine usage. Join the Community: Participate in Go forums and contribute to open-source projects. Additional Resources: The Go Programming Language Documentation Effective Go Go by Example A Tour of Go Happy Coding with Go!